Hello,
Iām Lucian (@lucianghinda on Twitter), and I am the curator of this newsletter.
Last week I launched Code Summaries, where I summarise technical articles about Ruby with code.
If you want to see a short version of this newsletter with only the content that has code, you can see it here. It is a presentation that has one tweet per slide uploaded to speakerdeck.com.
This edition was created with support from @adrianthedev from Avo for Ruby on Rails (a friendly full-featured Rails admin panel) and from @jcsrb, who sent me recommendations to include in the newsletter.
If you have any feedback or ideas about this newsletter, please reach out on Twitter or via email at hello@shortruby.com
š Xavier Noria shared a short explanation of how constants work in Ruby:
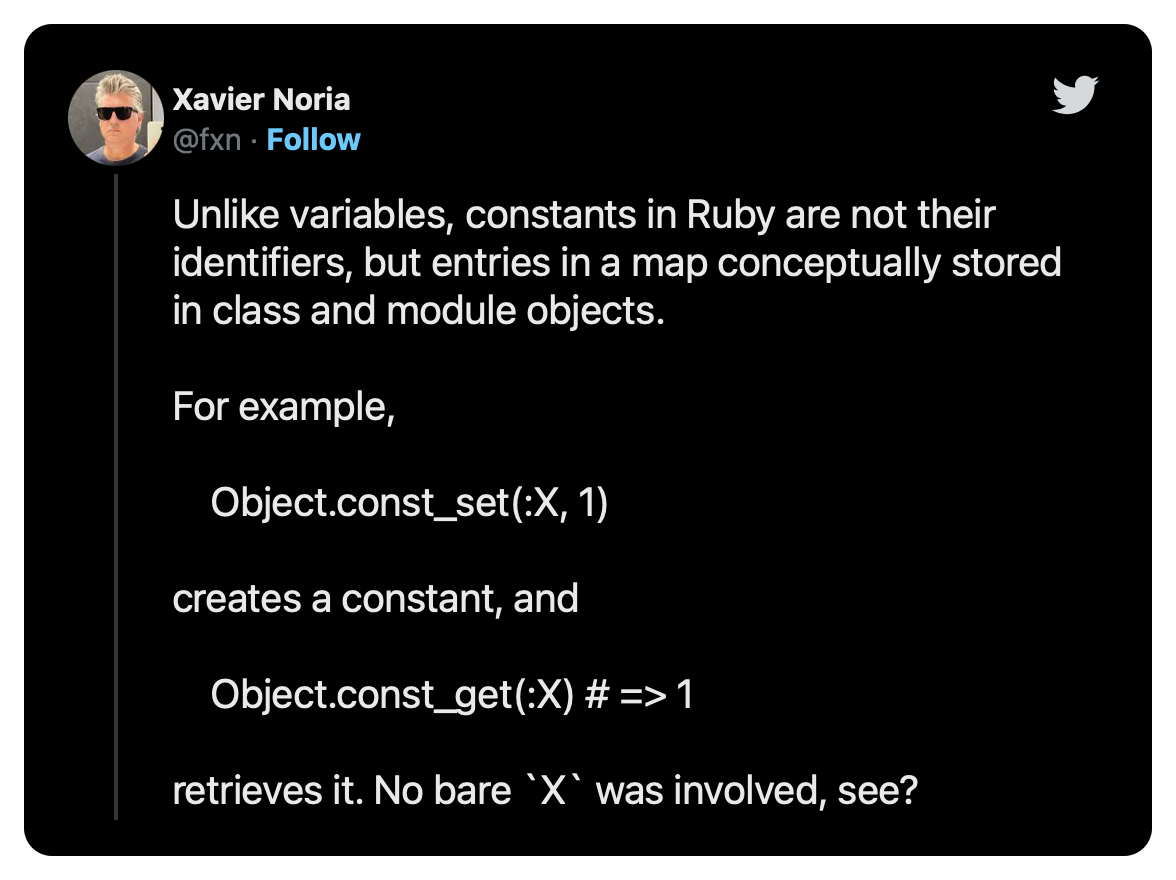
Constant identifiers and constant paths are just convenient interfaces into those maps. Helpful for common usage, and for giving the illusion of namespaces. The constants API gives a direct access to those maps, and allows other things like listing or deleting, for example. - Xavier Noria
š Thiago Massa shared a new example of pattern matching with hashes:
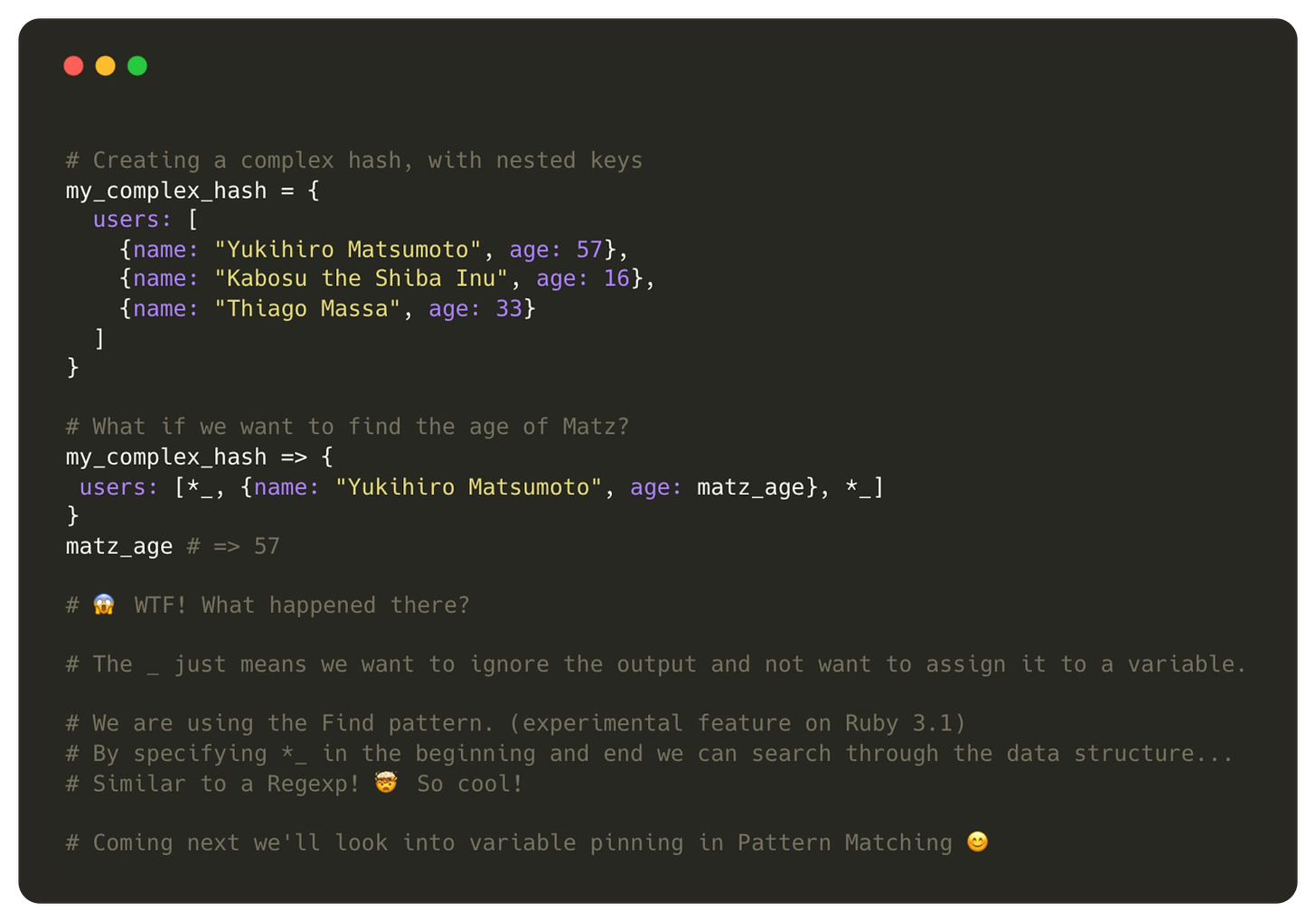
He also shared the gist in case you want to play with it.
š Shino Kouda shared a short code example of .map(&:to_s) and Brandon Weaver explain more in depth how this works:
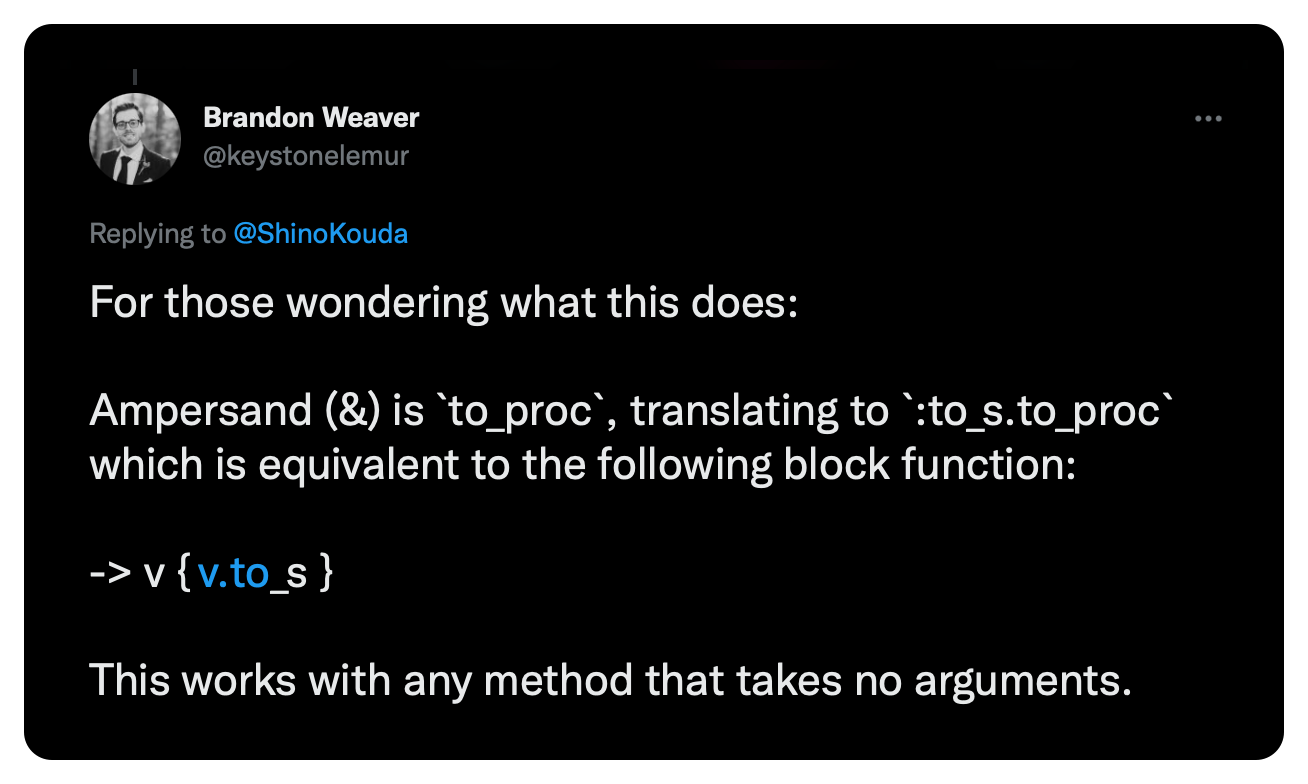
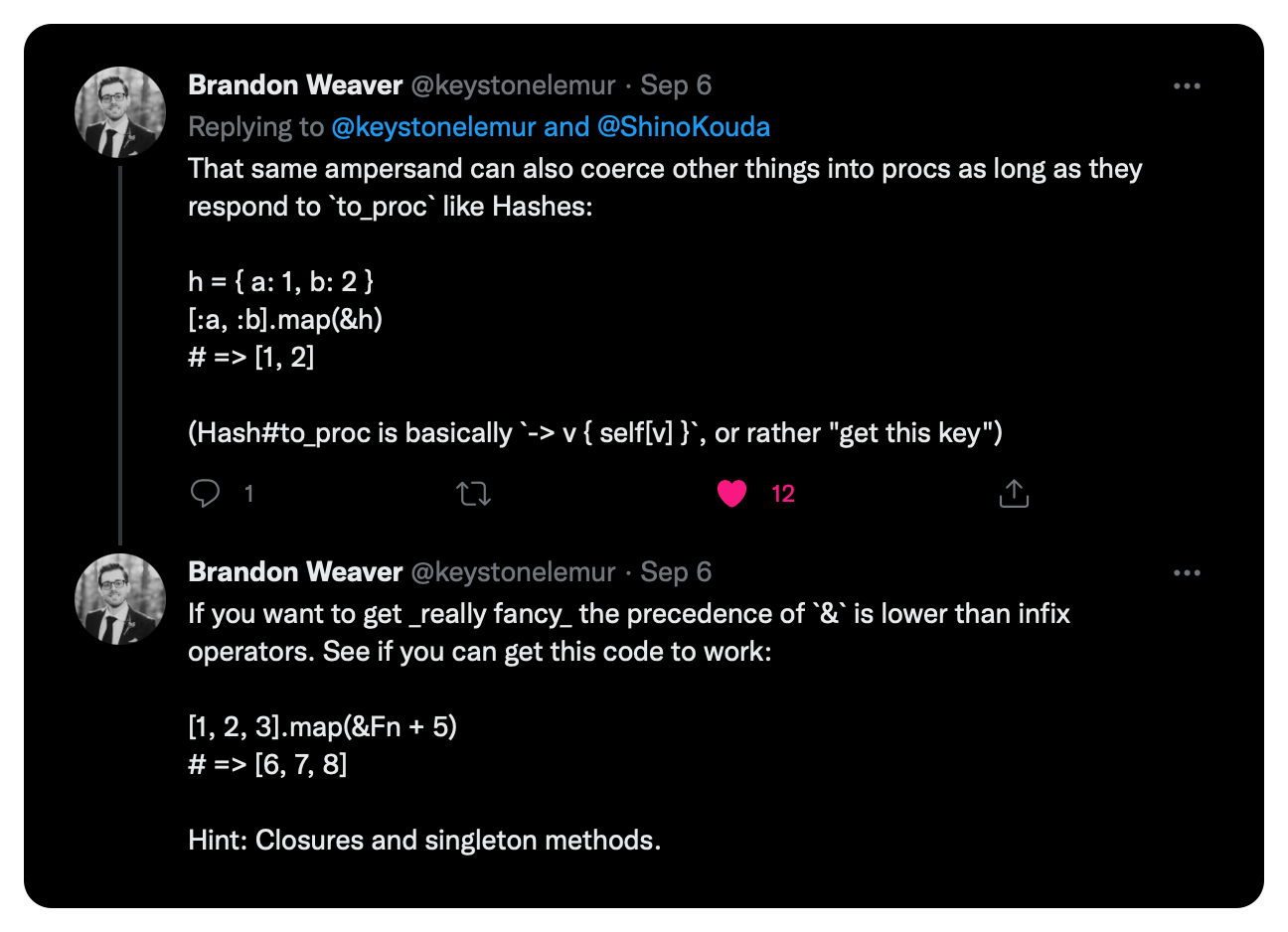
š Thiago Massa shared an example of how to use the Pin operator (^) pattern matching:
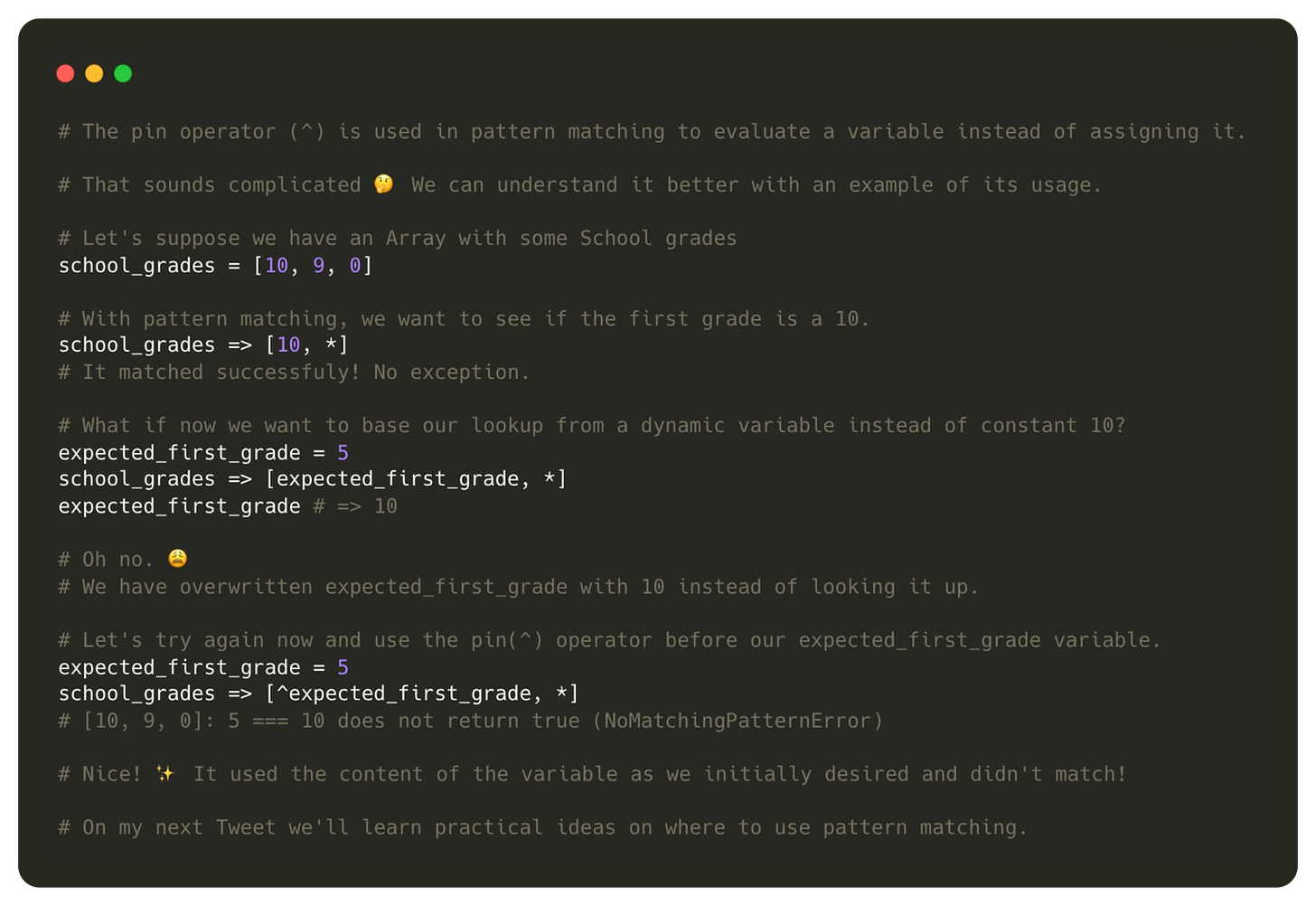
š Joel Drapper shared an example of another underused operator, XOR:
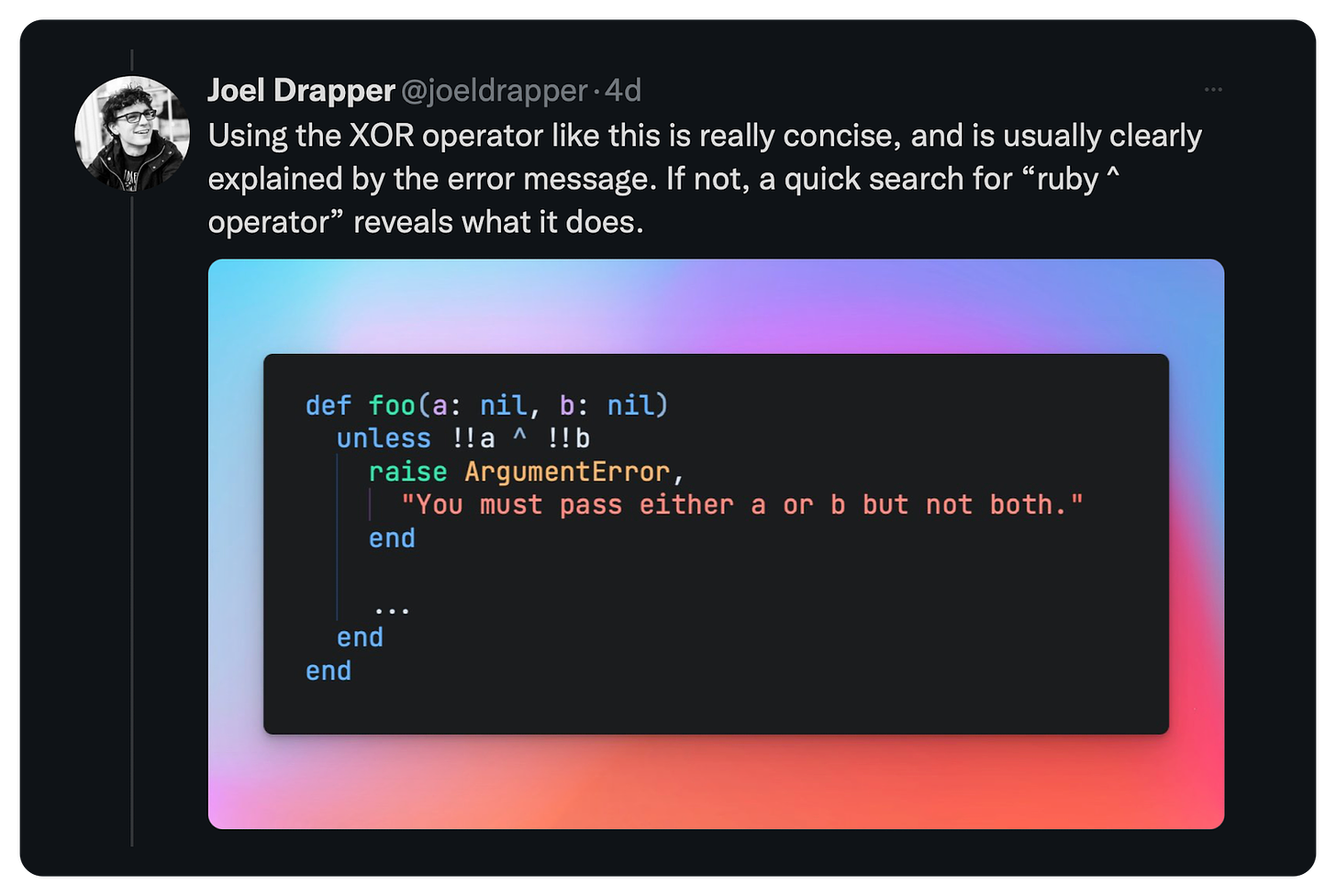
š Lucian Ghinda shared another example of using XOR:
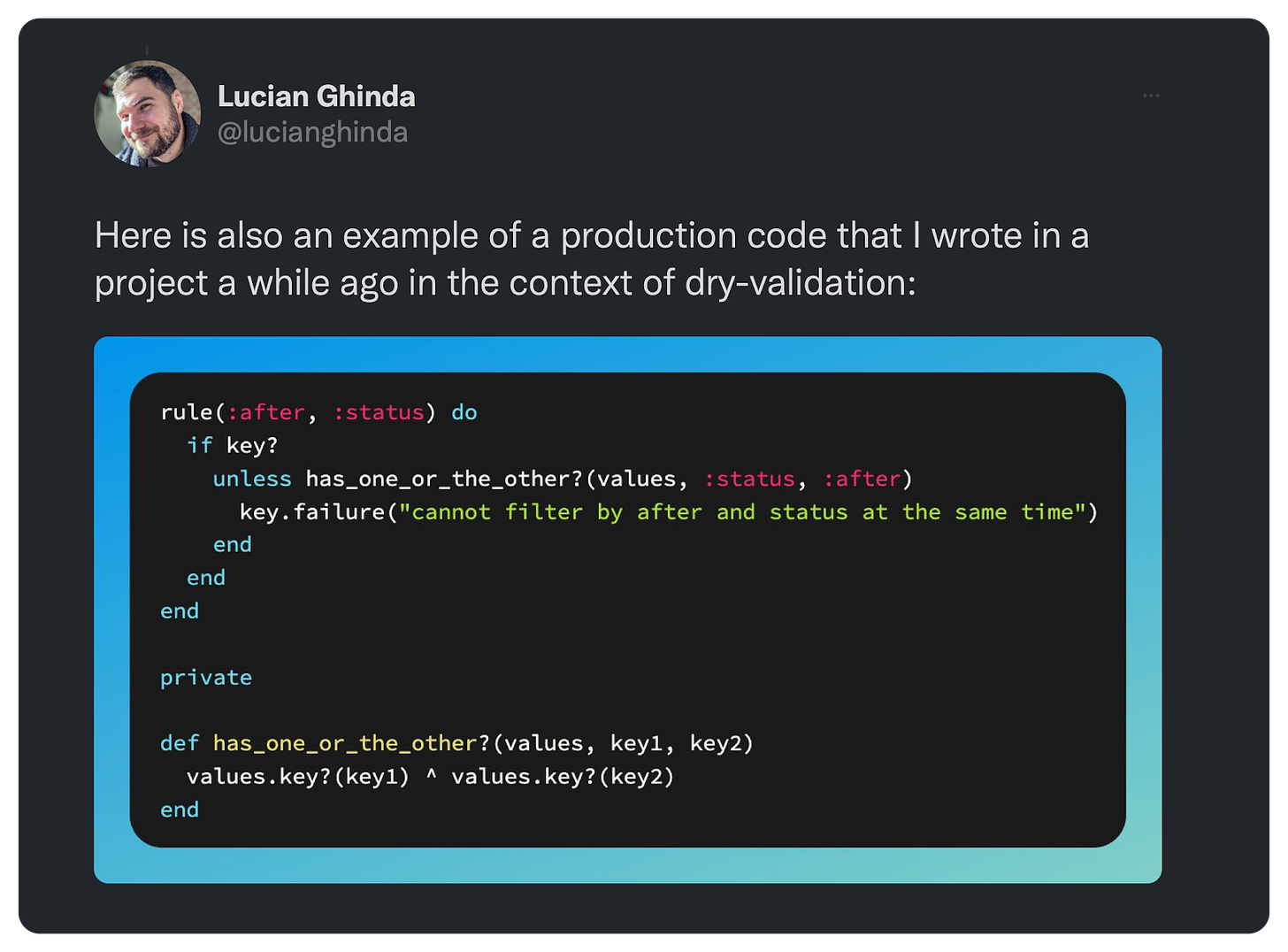
But you should read the entire thread, as it has some excellent discussions about using these operators.
š Kirill Shevchenko shared two code samples explaining how |= is creating a new array instead of adding to the existing one:
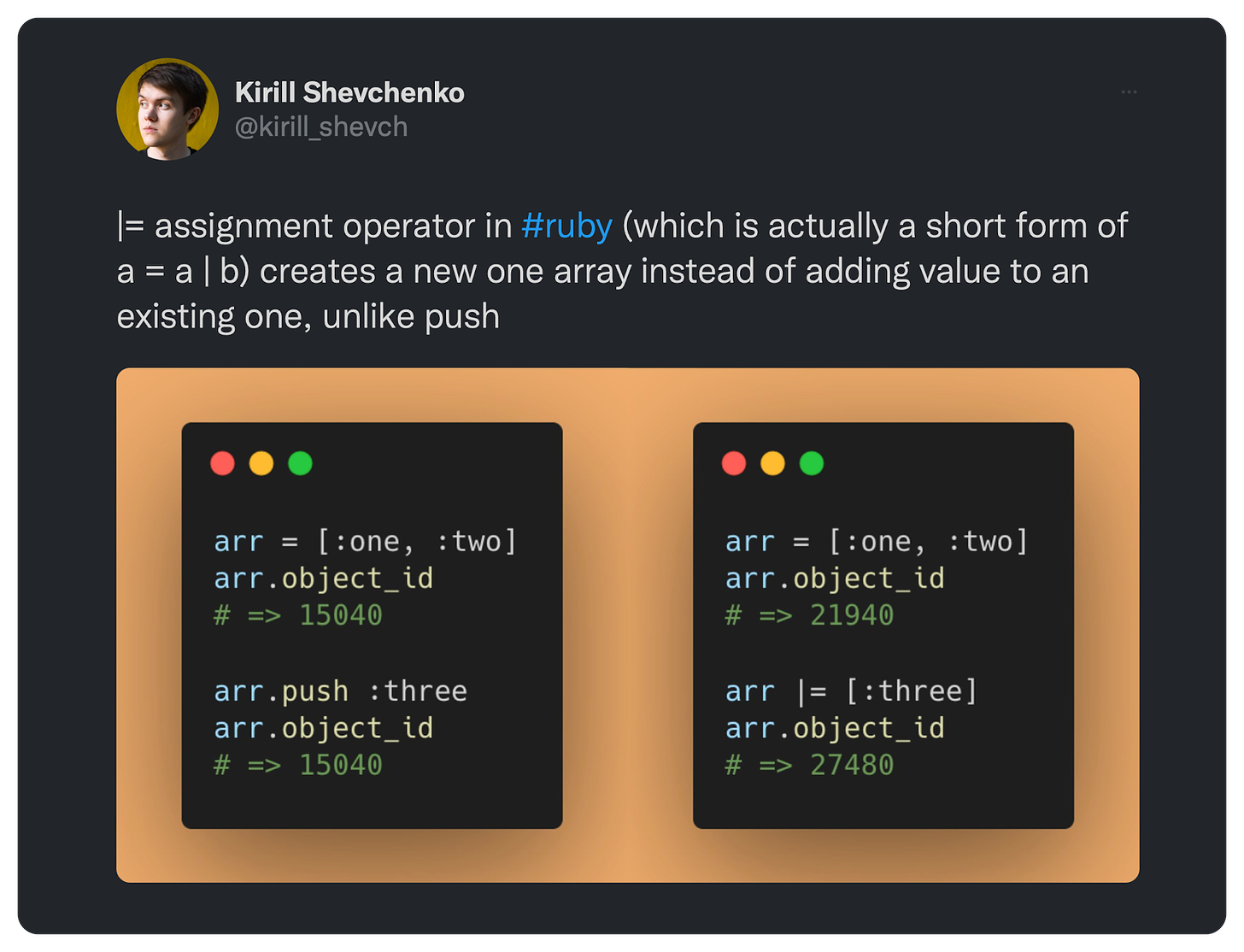
š JoĆ«l Quenneville shared code about how to generate infinite series with Enumerator:
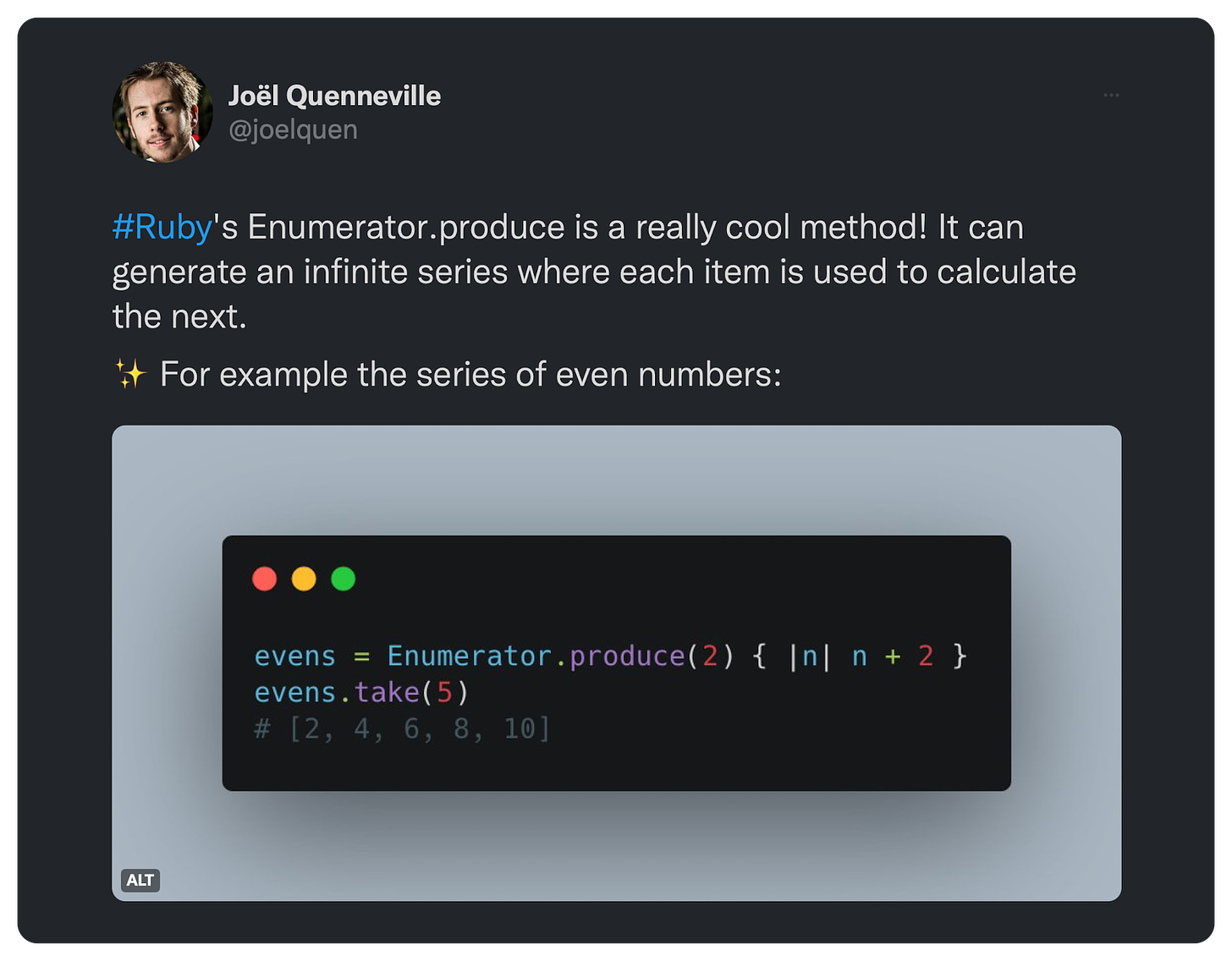
As the produce cannot be used to generate series where the input from each step needs the output of the previous step, then he showed how to add unfold to the Enumerator to achieve more:
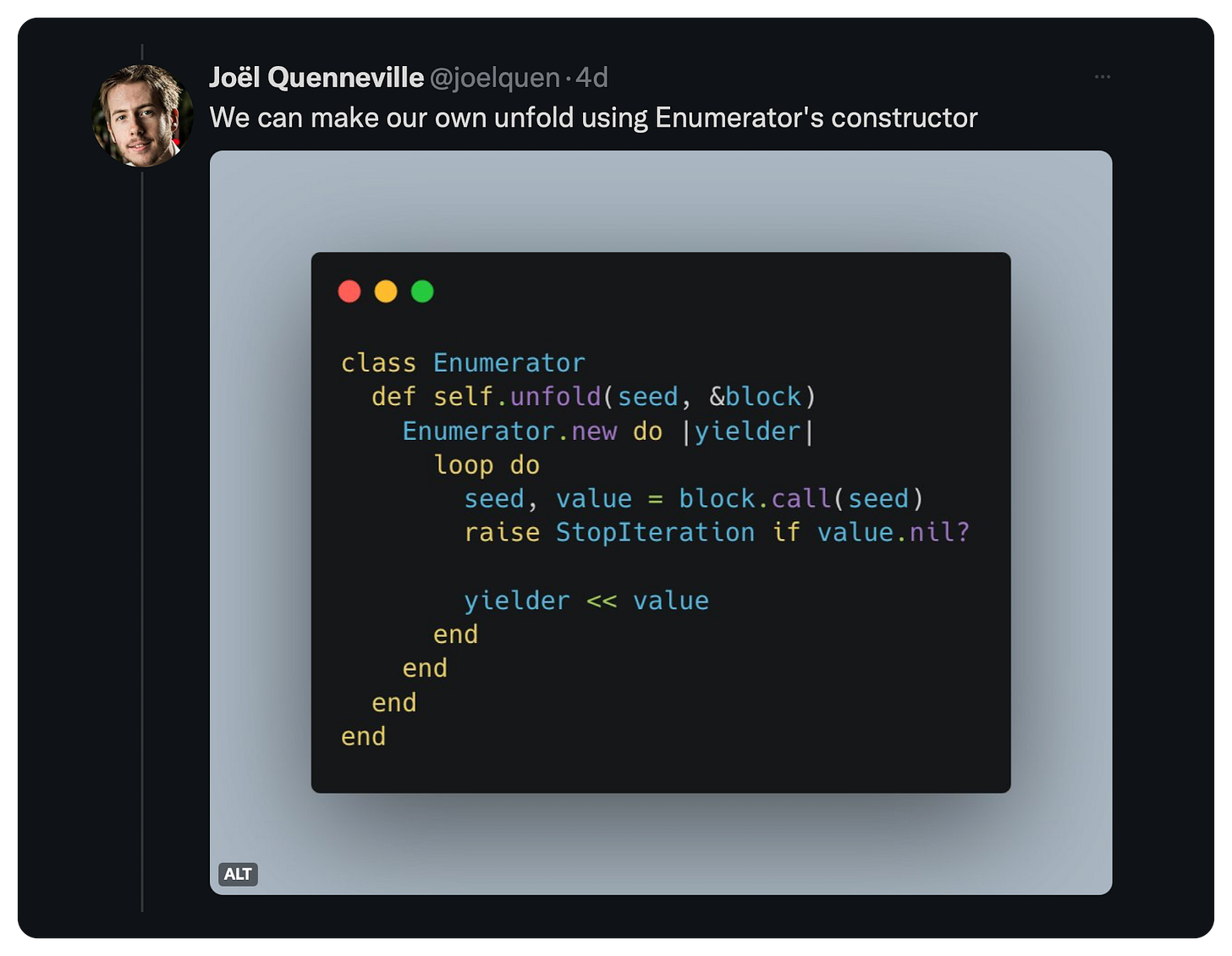
And here is how he showed how to use that:
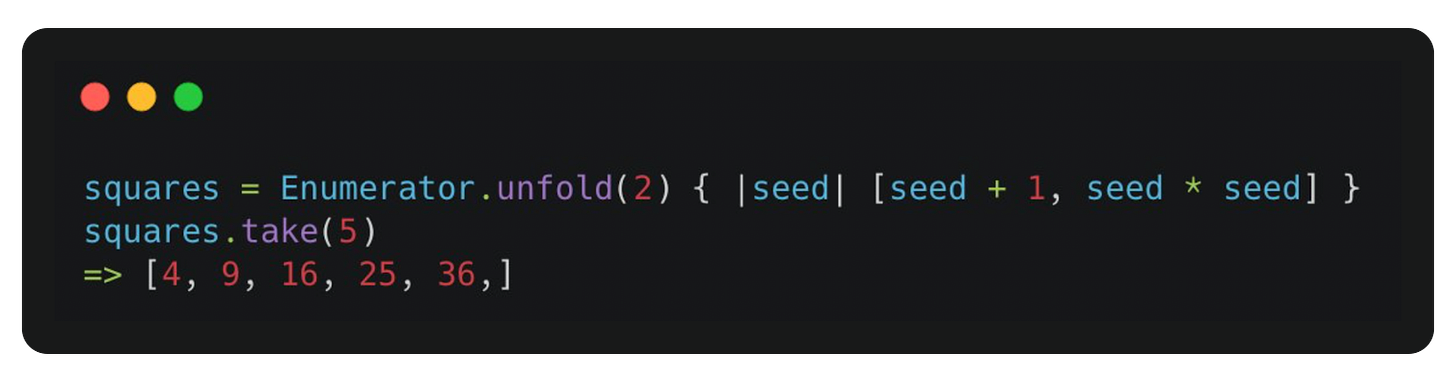
There are more examples shared in that thread.
š Lucian Ghinda shared a thread about an opinionated way to learn Ruby:
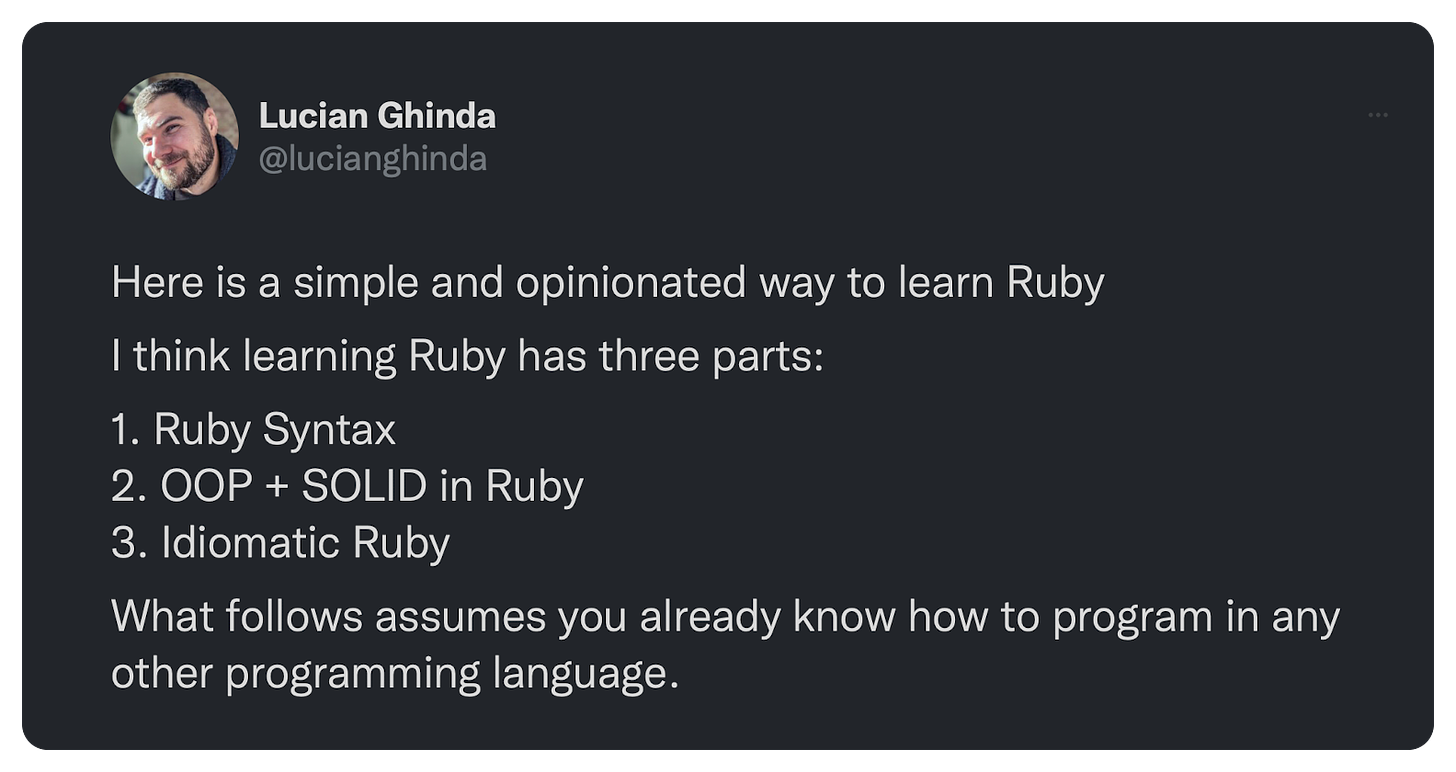
š Emmanuel Hayford asked a smart question: How to explain Class.class being Class:
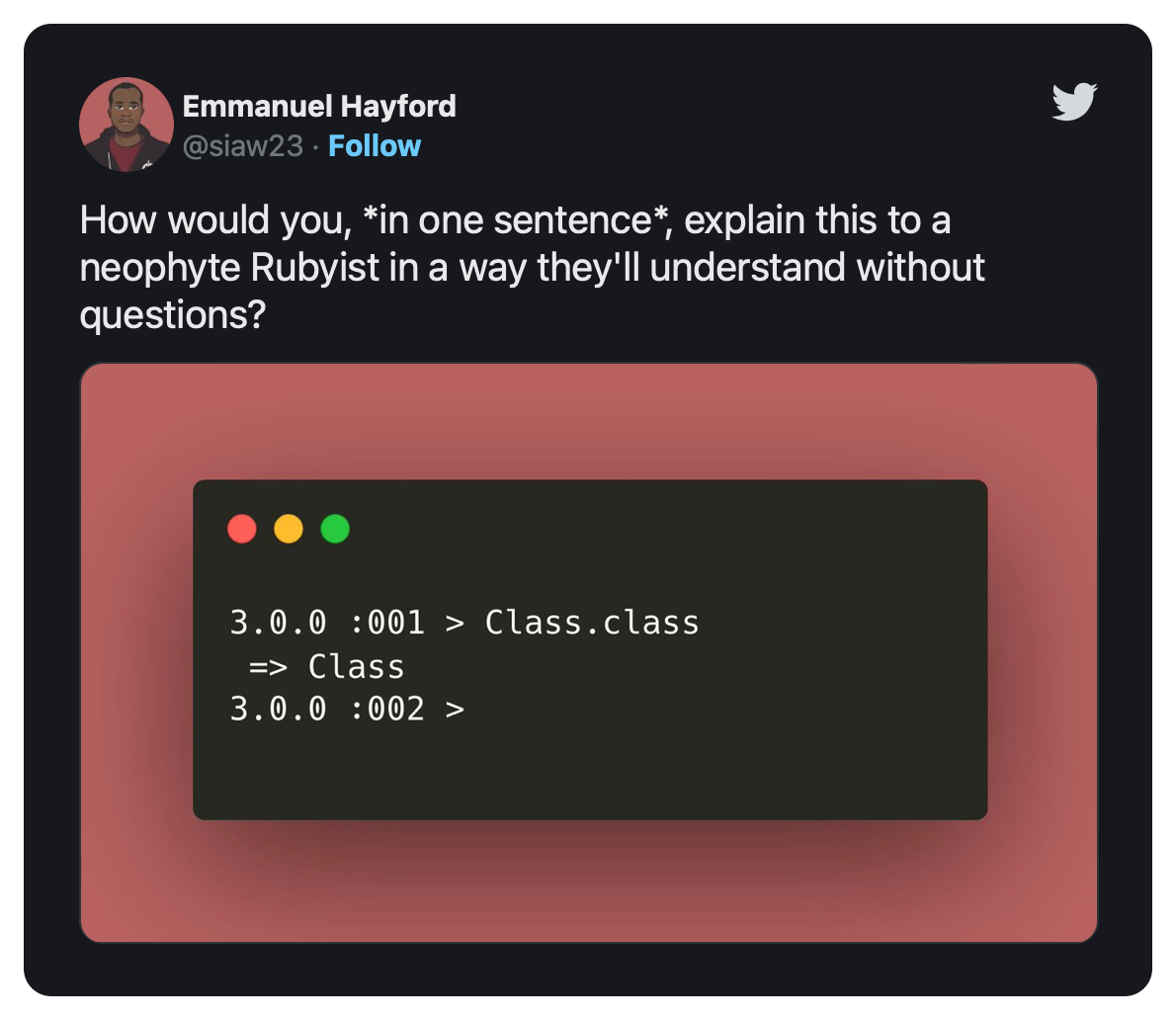
Here are some of the examples shared in that thread by Brandon Weaver, Lucian Ghinda, John Cheek, Ariel Caplan, Eric Halverson:
š Brandon Weaver shared an experimental piece of code to implement proc_line:
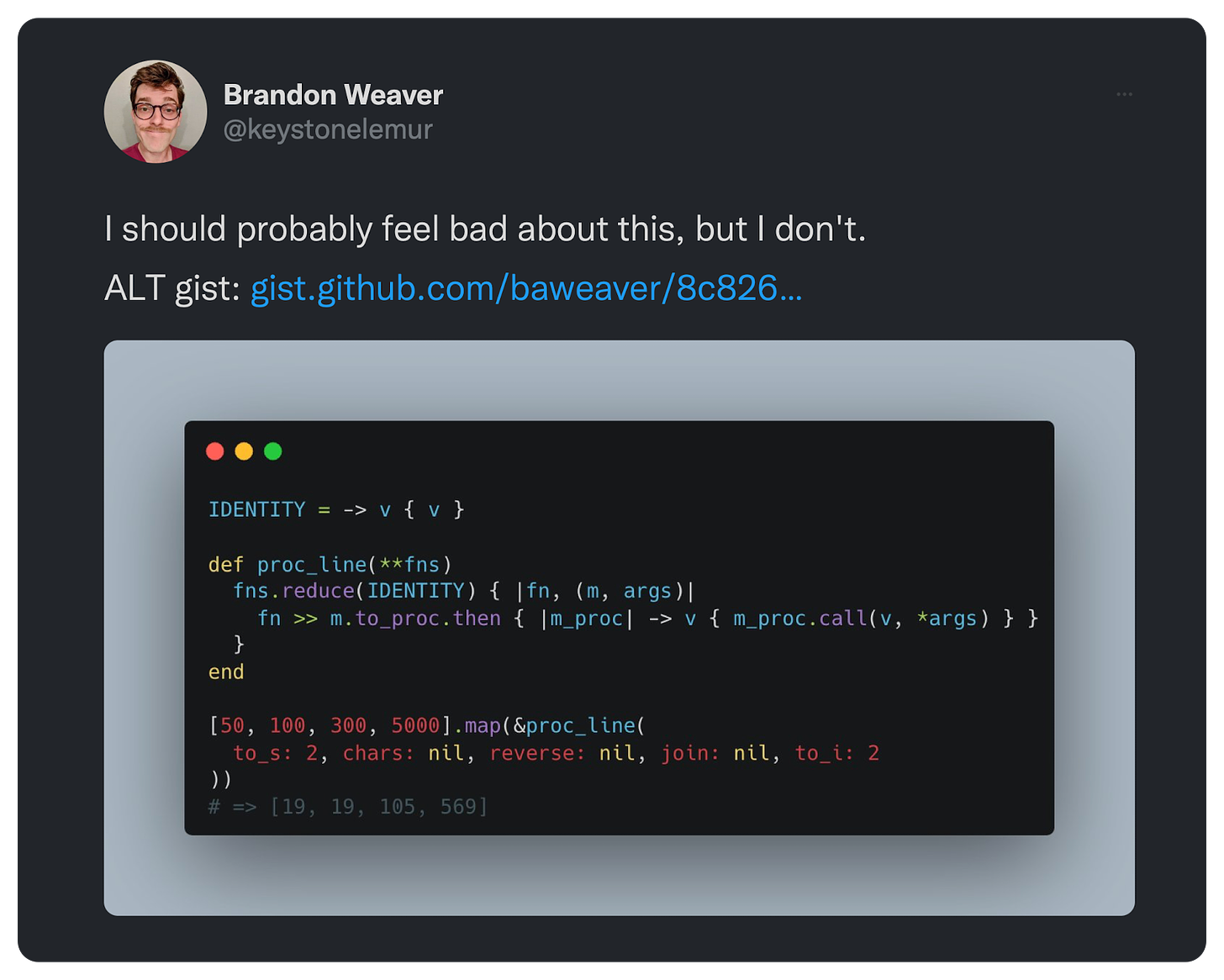
š Joel Drapper shared an example of how to use Phlex with ERB:
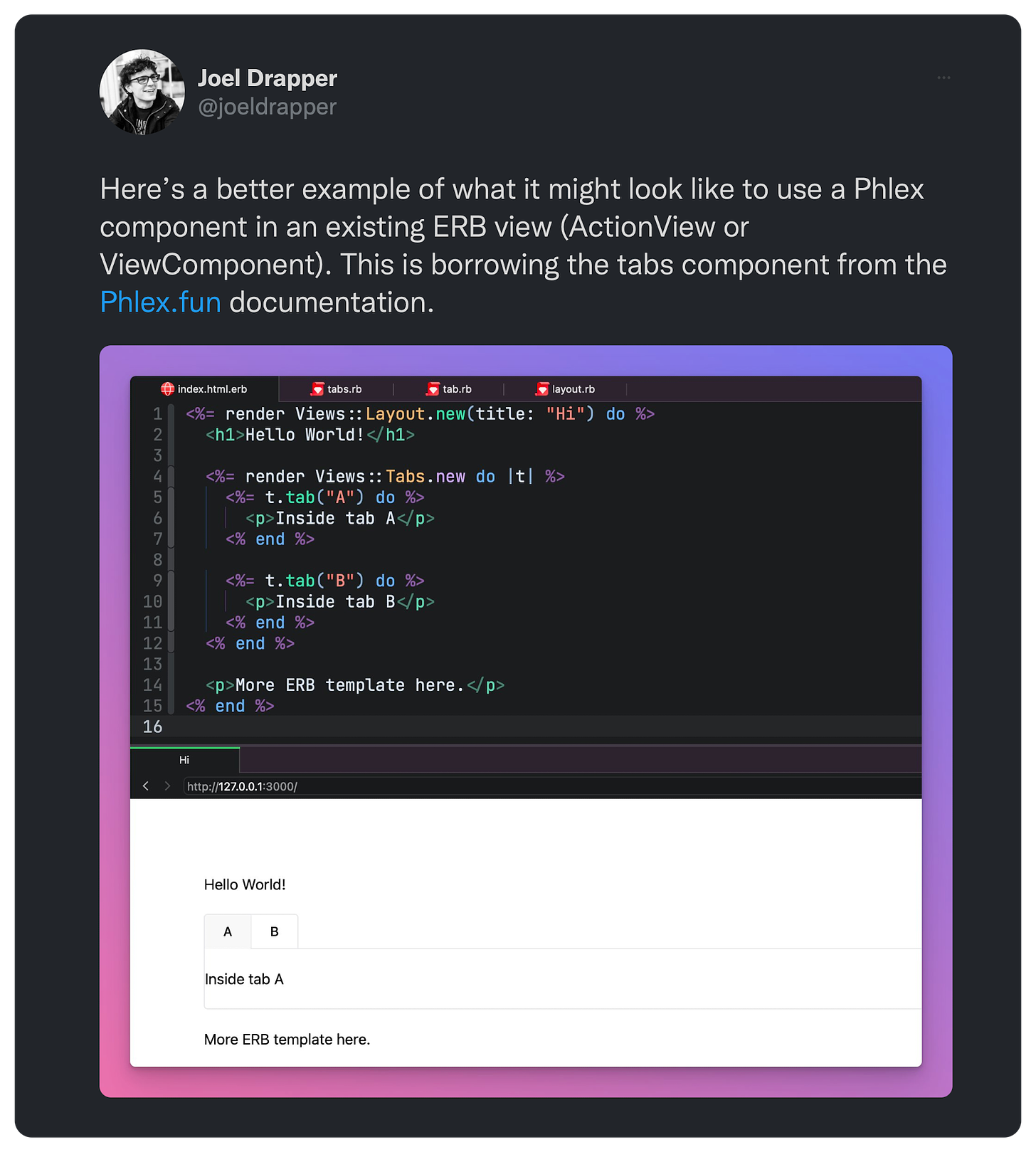
š Jean Boussier shared their process to improve performance on string interpolation. Amazing read!
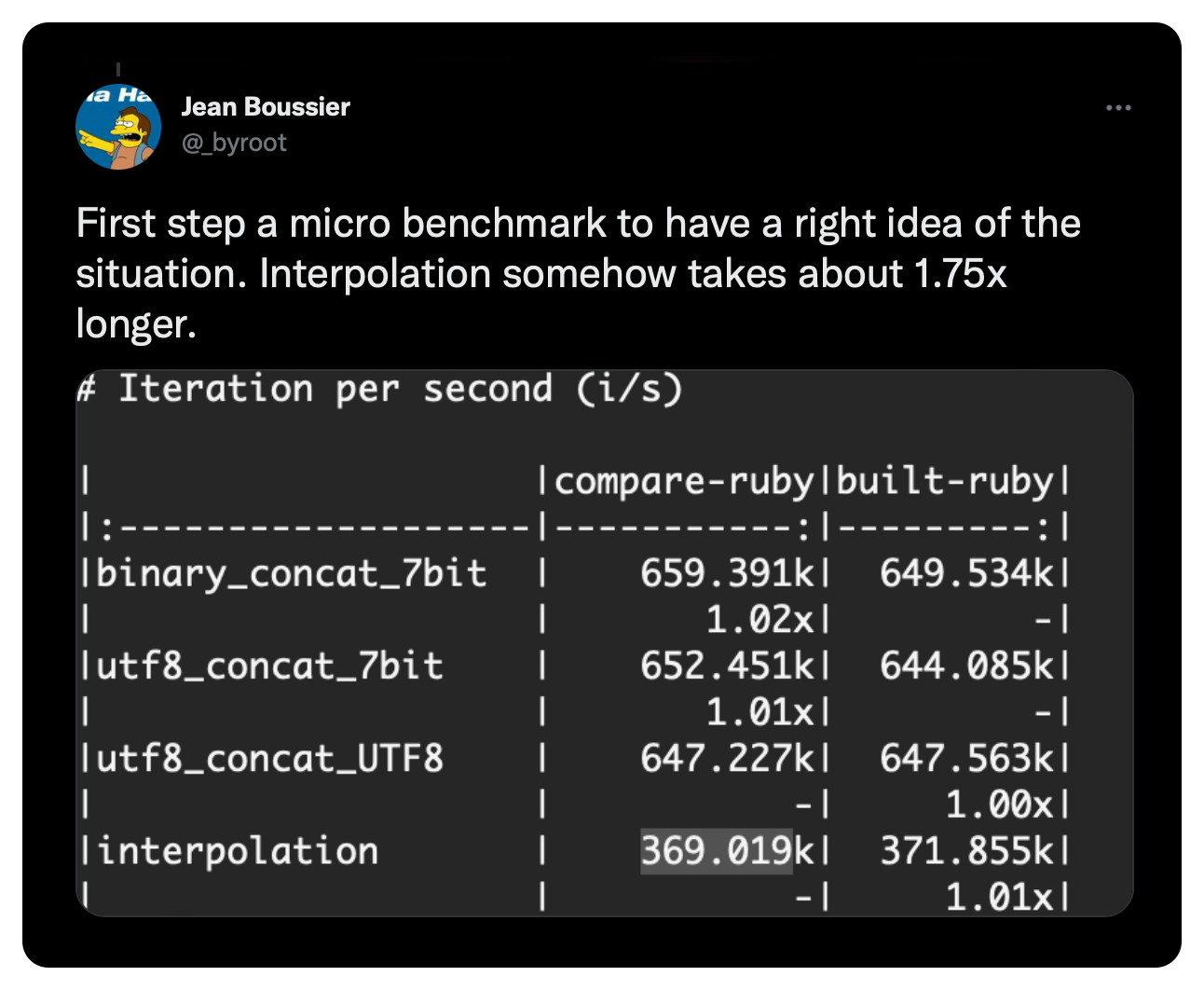
š Kevin Newton shared a quick command to help understand Ruby TRICK entries published by Yusuke Endoh here:
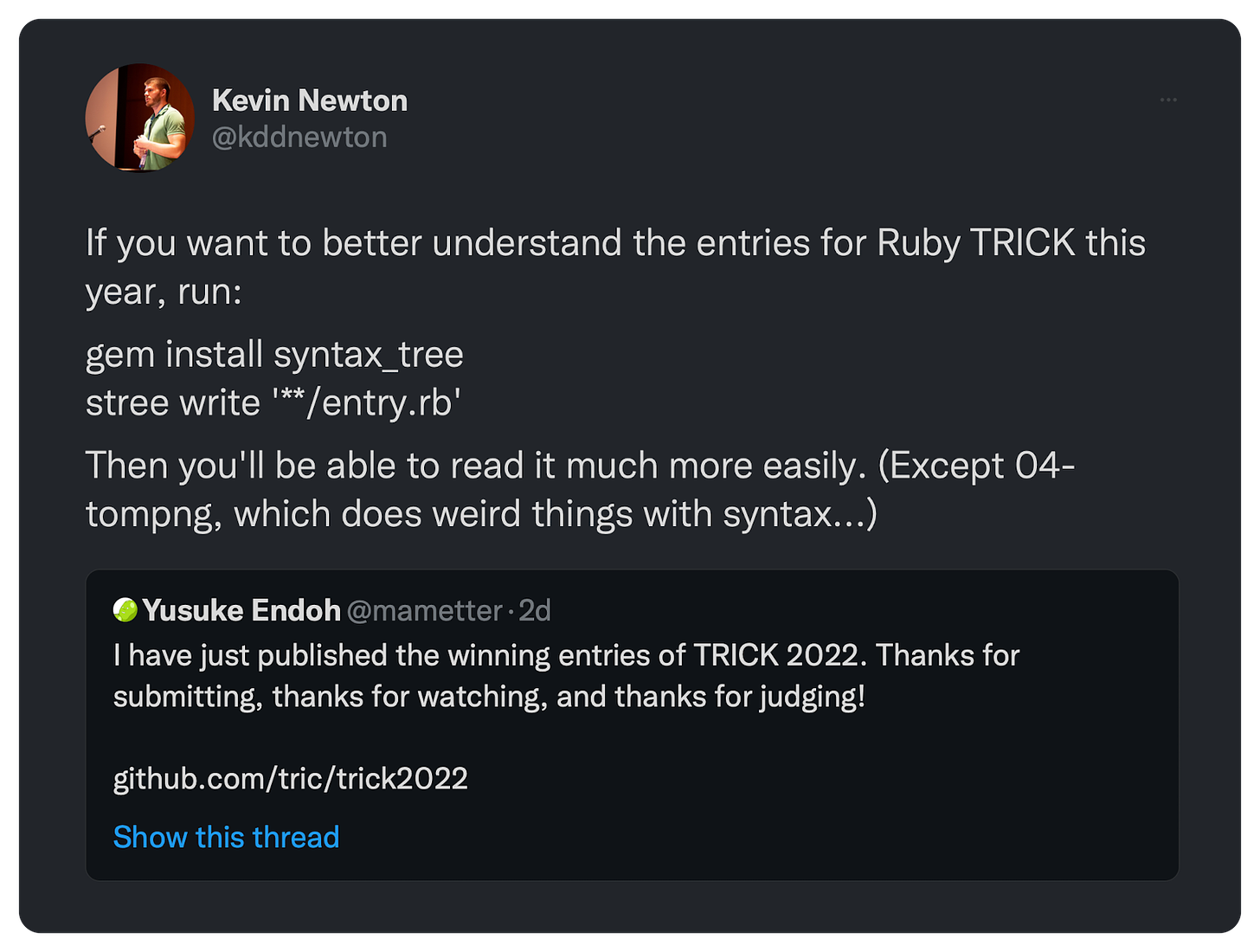
š Robby Russell asked what is one main improvement for speeding up tests and received some very good answers.
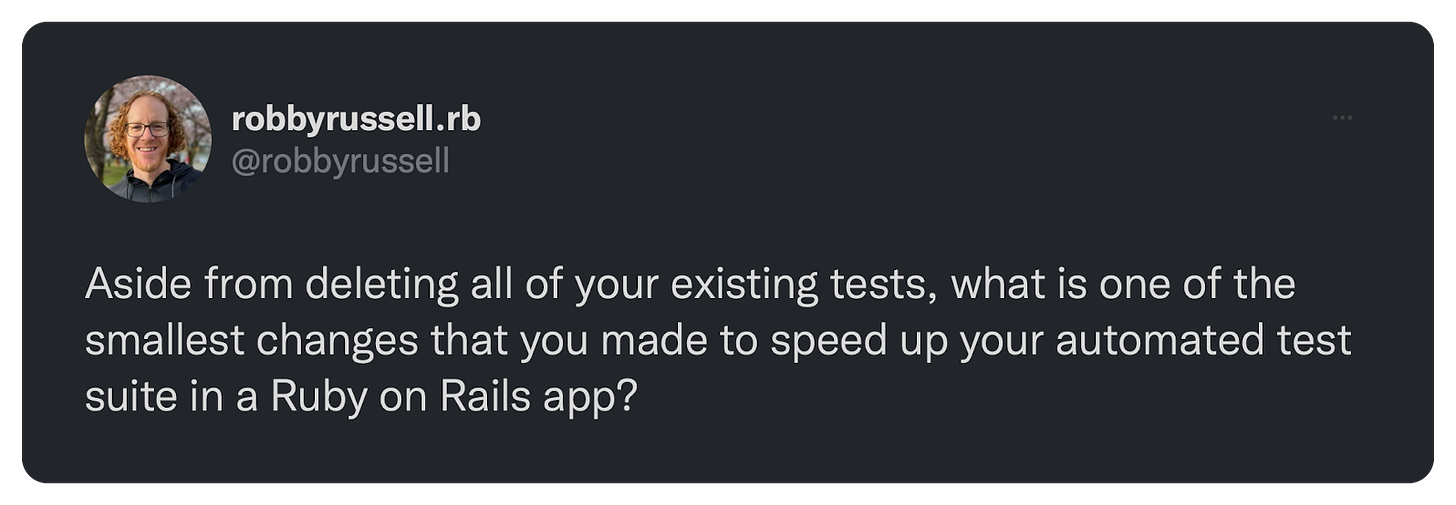
Here are some of the responses shared by Brandon Weaver, Greg Navis, Konnor Rogers, Andrey Novikov, Benjamin Silva H, Andrew Ek, but please do go and read the entire conversation:
š Thiago Massa asked a question and shared a code sample about the ampersand Ruby idiom:
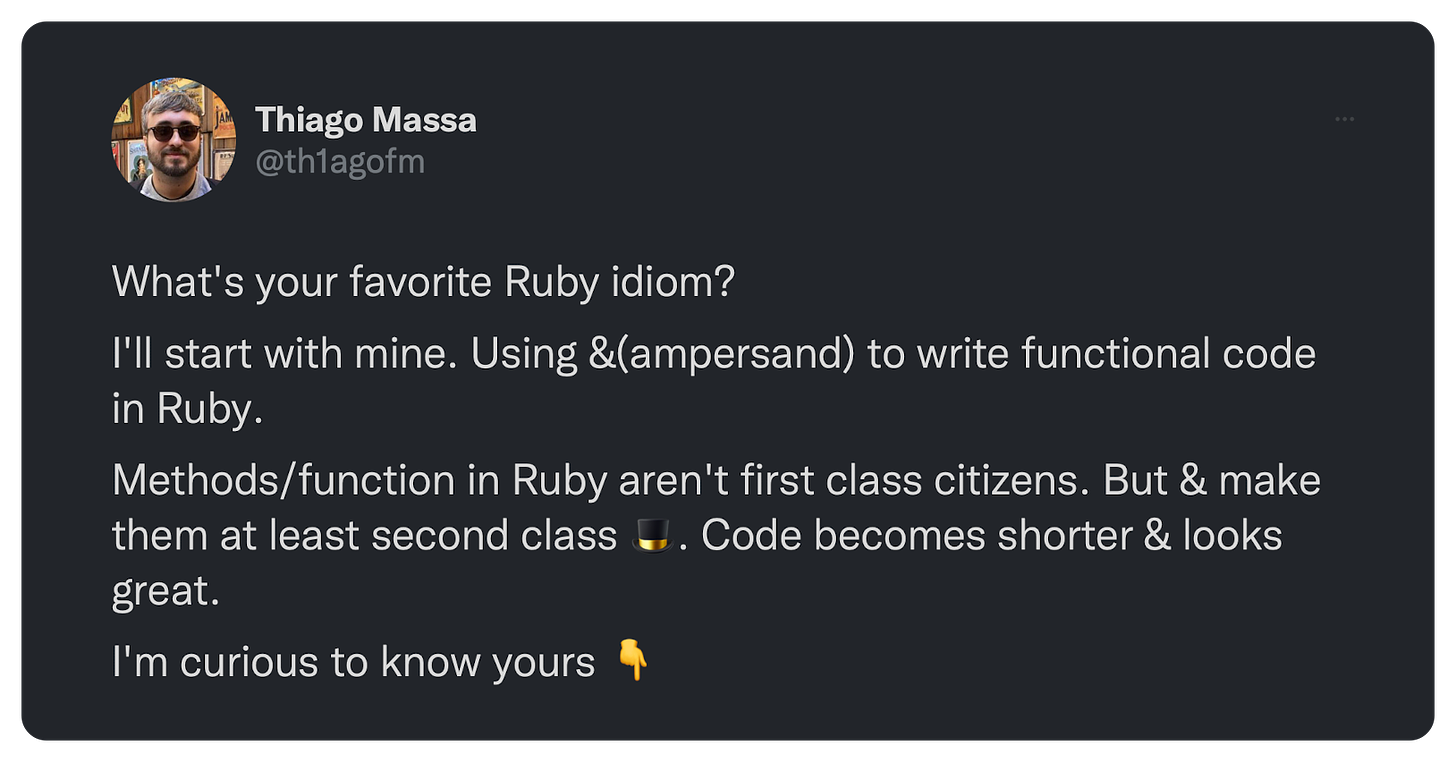
And along with this question Thiago shared a code sample:
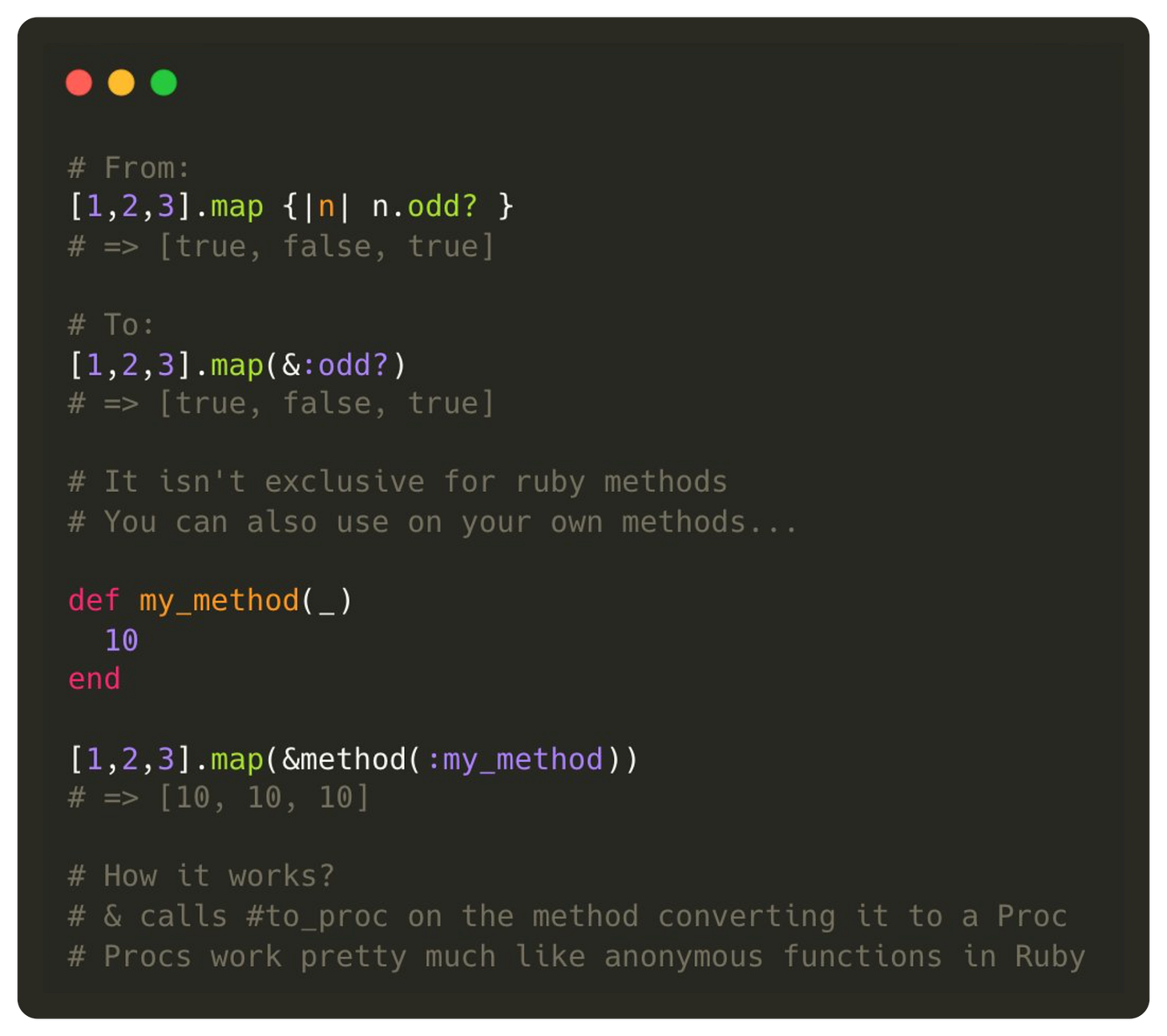
He received some inspiring responses, read the entire conversation. Here are two of them:
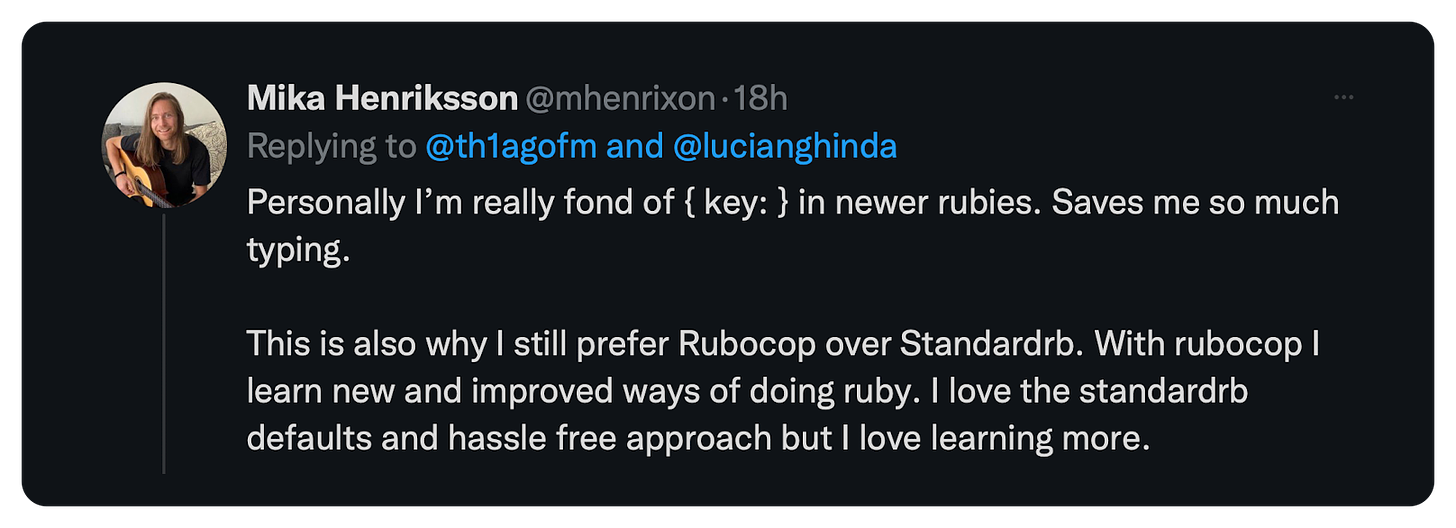
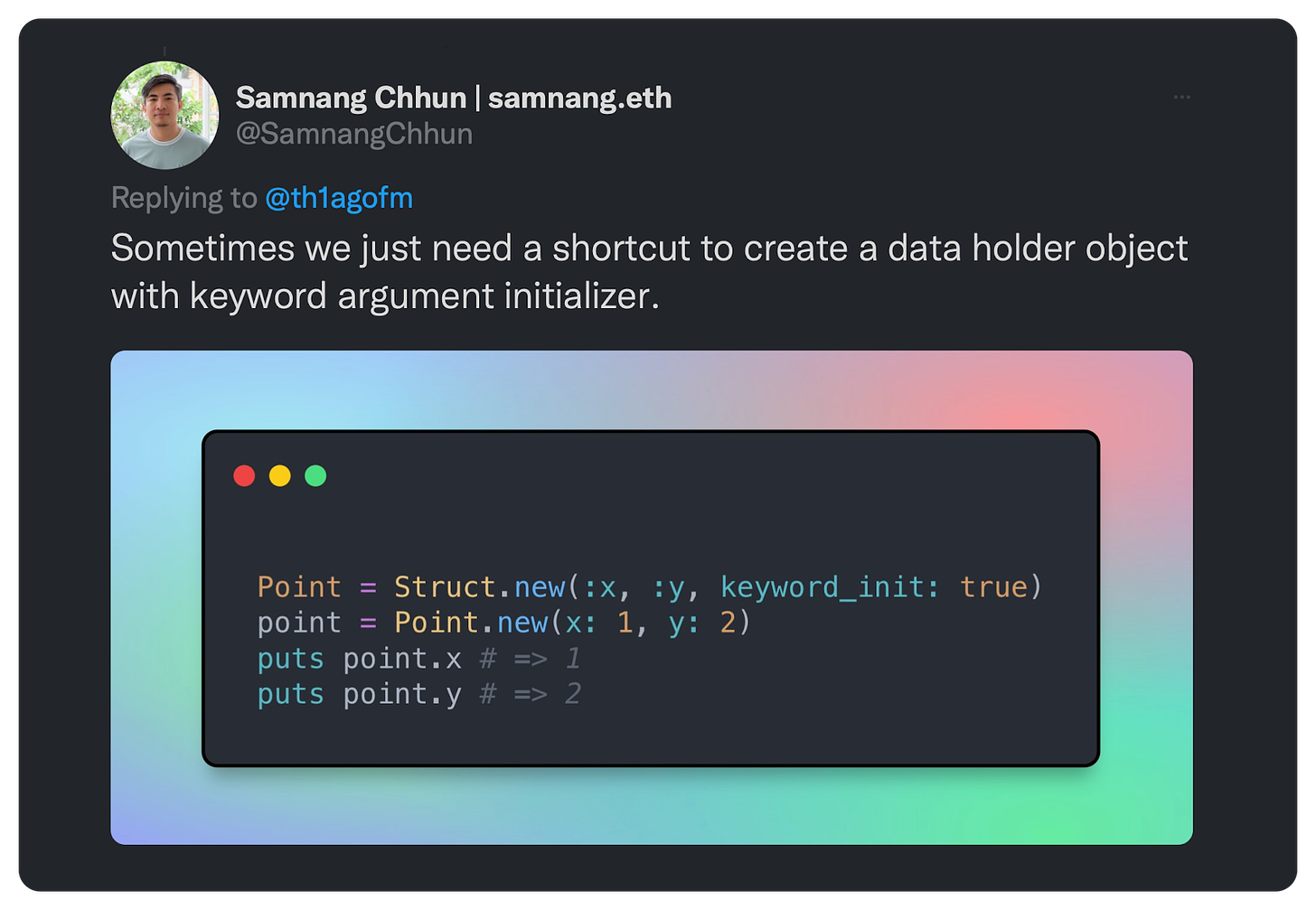
š Stan Lo shared a list of feature proposals for Ruby debug:
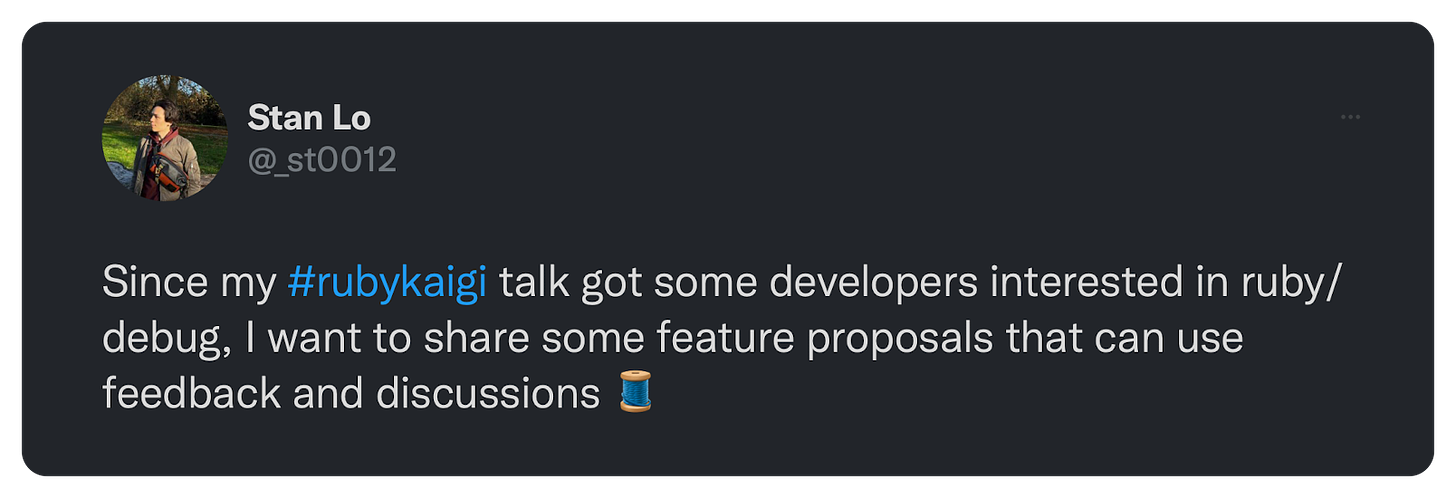
š Joel Drapper shared a new update for Phlex.fun showing support for conditional CSS classes:
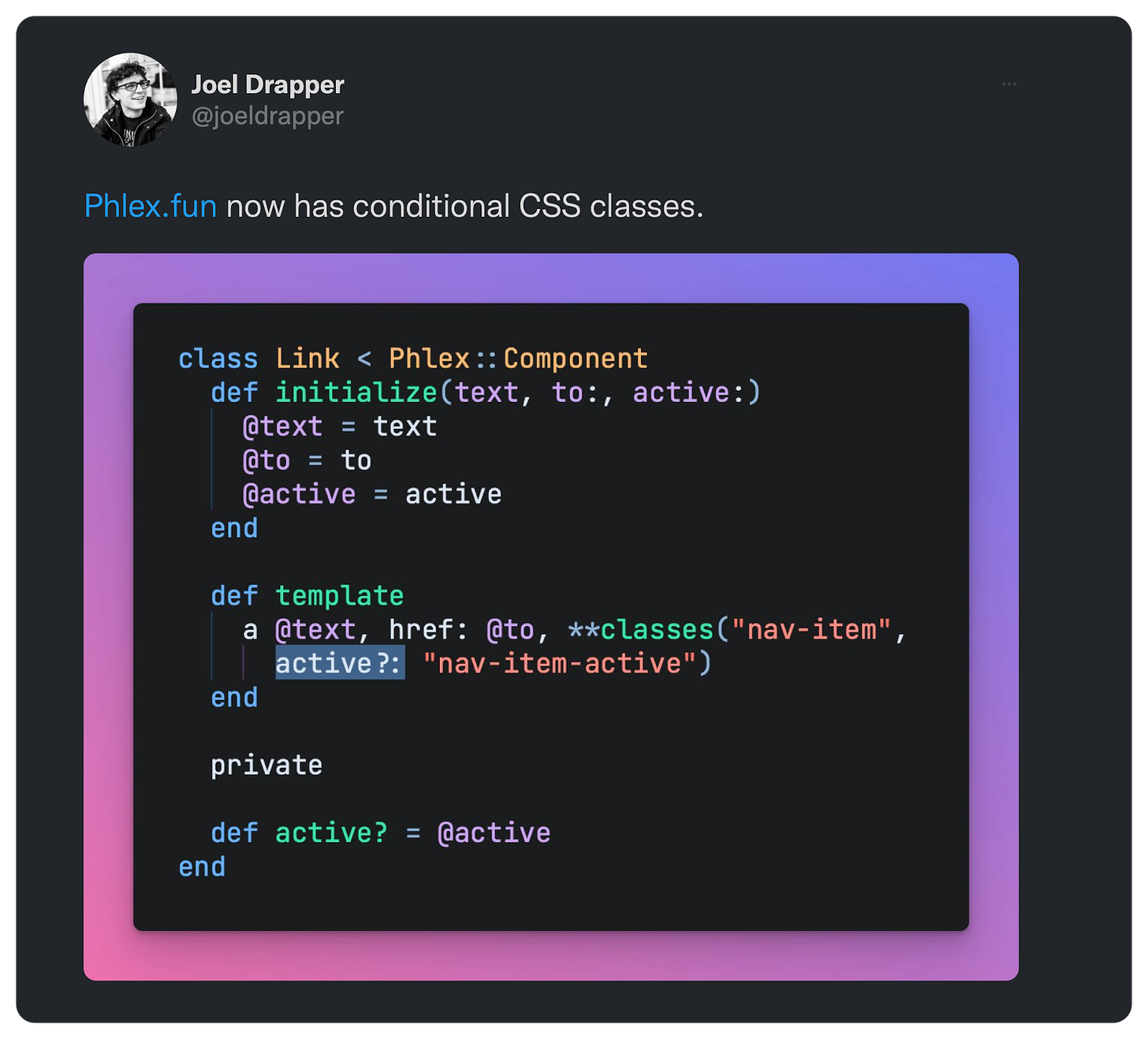
š Cj Avilla shared code showing how to use right assignment / pattern matching:
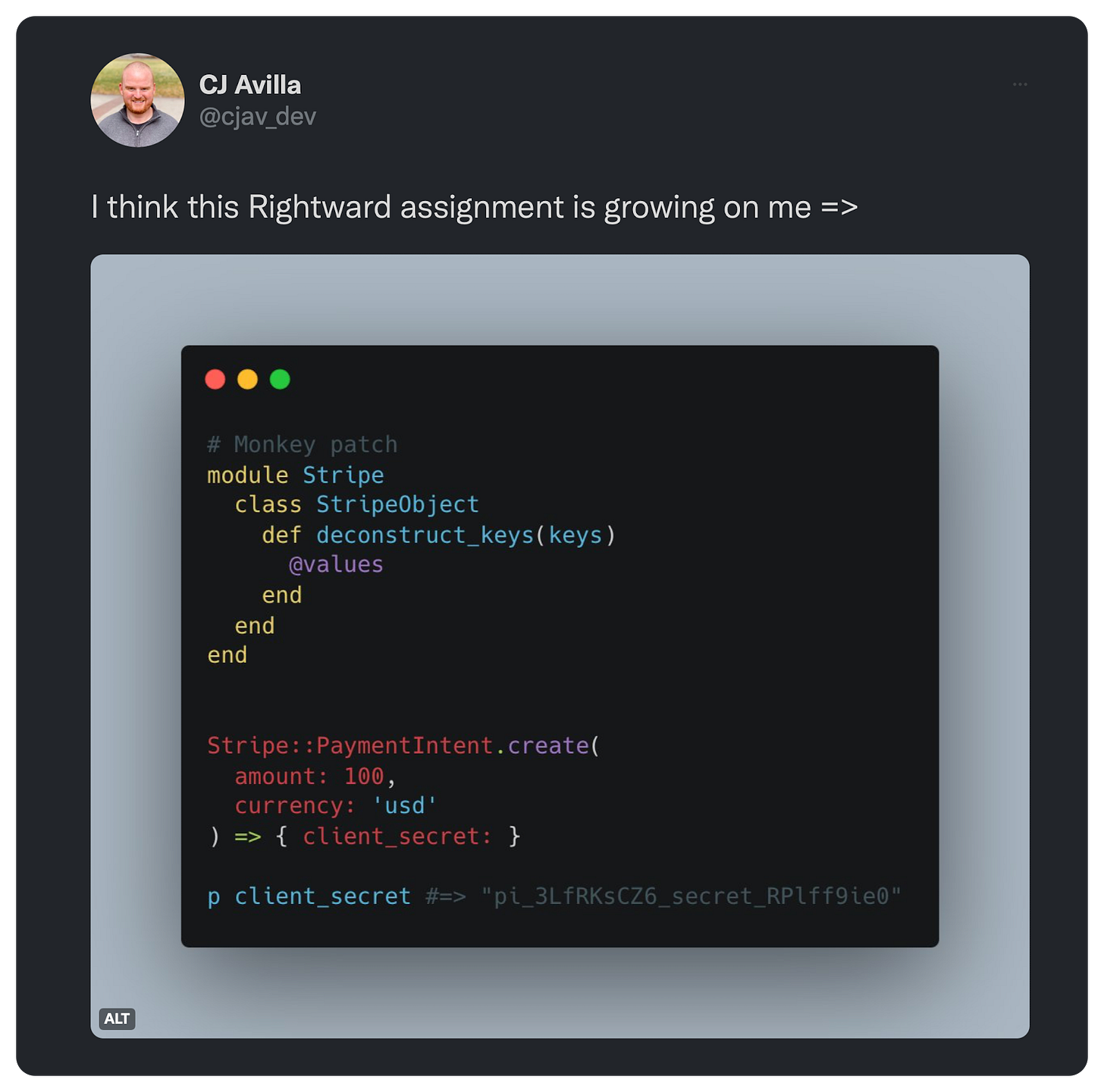
š Matt Swanson shared code about how to use modules to organize features:
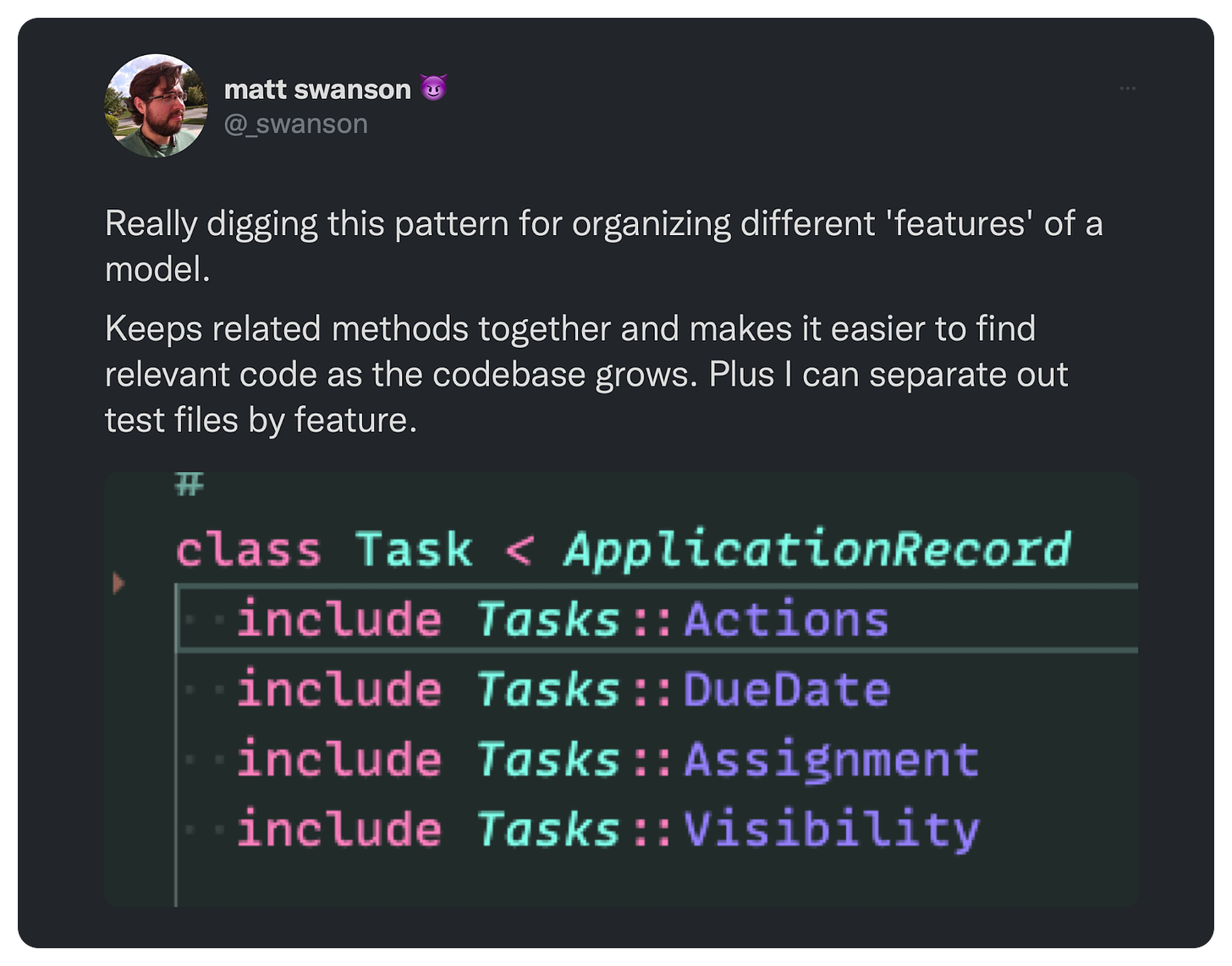
Here are some interesting replies, but I really recommend you to read the entire conversation (for people not using Twitter use this)as it has great sugestions and some great explanation for various styles:
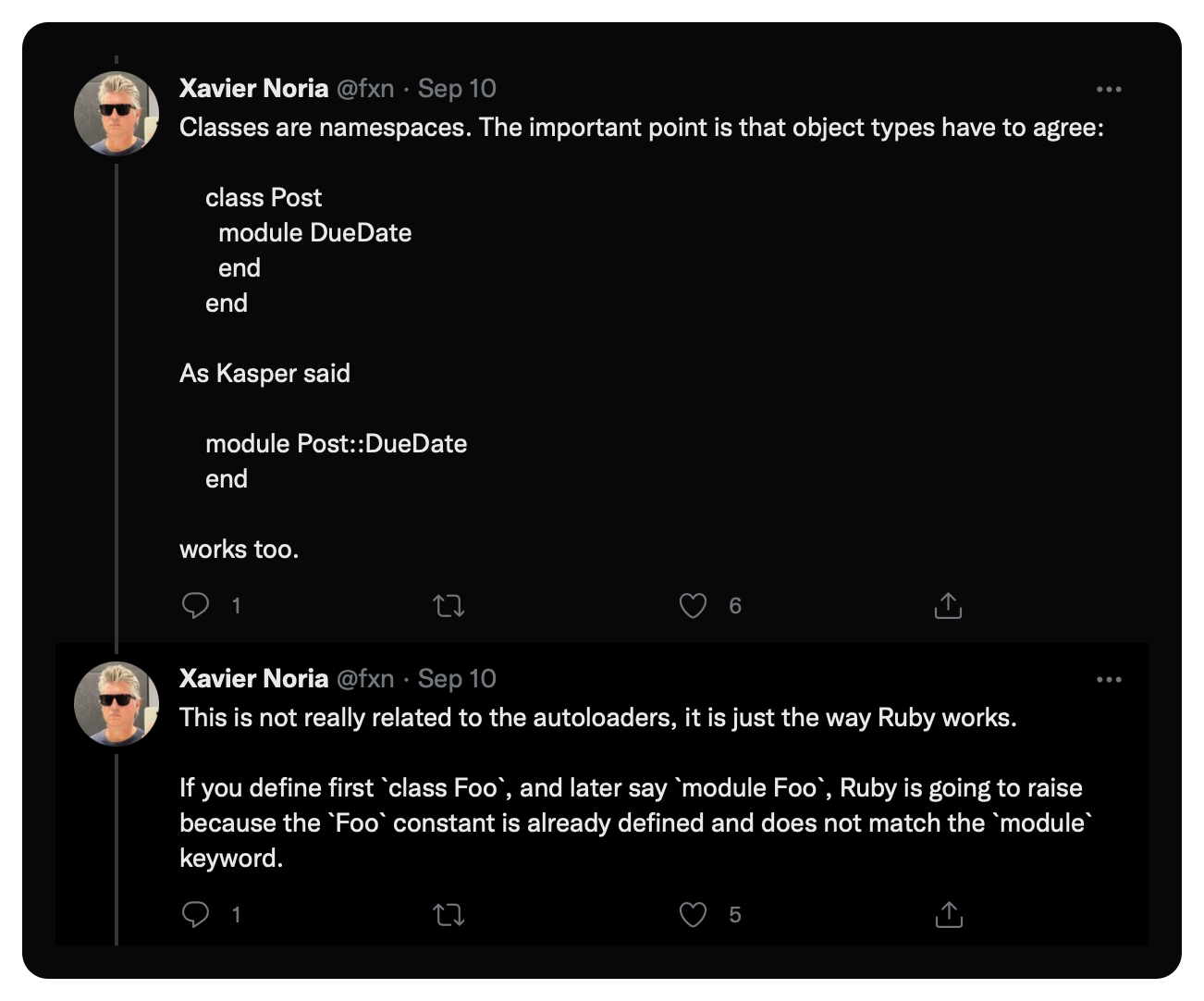
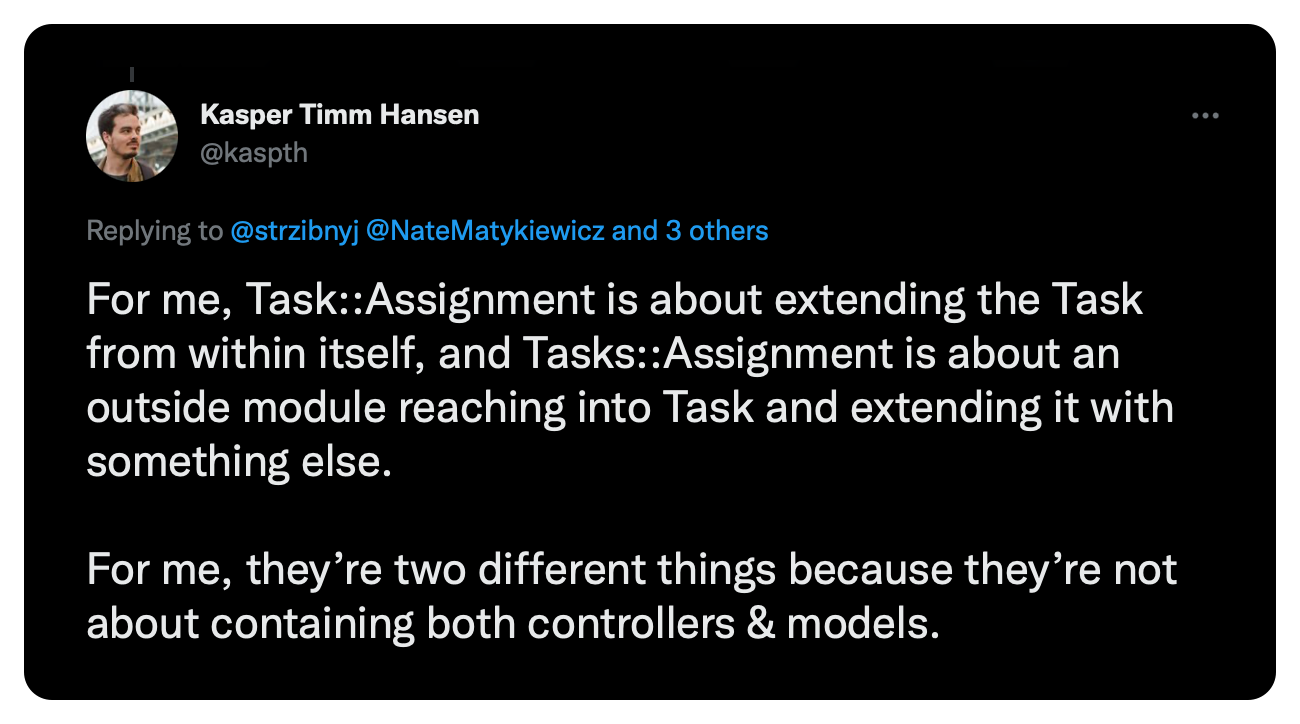
šShino Kouda asked what is the result of executing the following code:
Brandon Weaver shared explaining how [] works for arrays and hashes:
![`[]` is a method, `something.[](index)`, so the result would be `"a"`. This is similar to other operators like `1 + 2` is actually `1.+(2)`. That said, not common use, `dig` or `fetch` or `slice` would be more common if you're avoiding `[]` directly. `[]` is a method, `something.[](index)`, so the result would be `"a"`. This is similar to other operators like `1 + 2` is actually `1.+(2)`. That said, not common use, `dig` or `fetch` or `slice` would be more common if you're avoiding `[]` directly.](https://substackcdn.com/image/fetch/w_1456,c_limit,f_auto,q_auto:good,fl_progressive:steep/https%3A%2F%2Fbucketeer-e05bbc84-baa3-437e-9518-adb32be77984.s3.amazonaws.com%2Fpublic%2Fimages%2F50cc8860-6f2c-4d32-8dfc-720eaba904ed_1308x772.png)
š Kirill Shevchenko shared a code sample about how to use lazy enumerators:
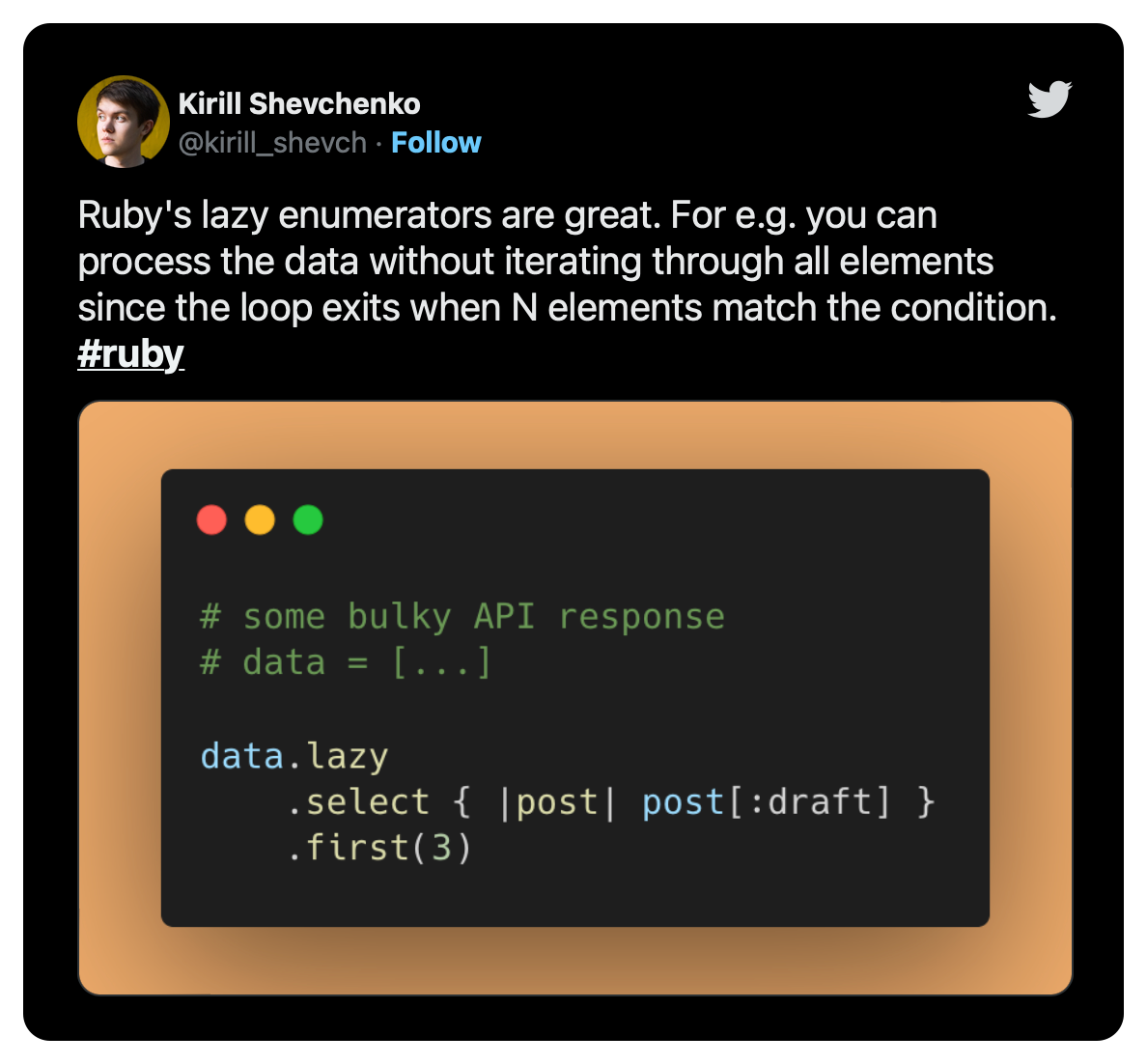
š Collin shared a tip about how to require a library when starting IRB:
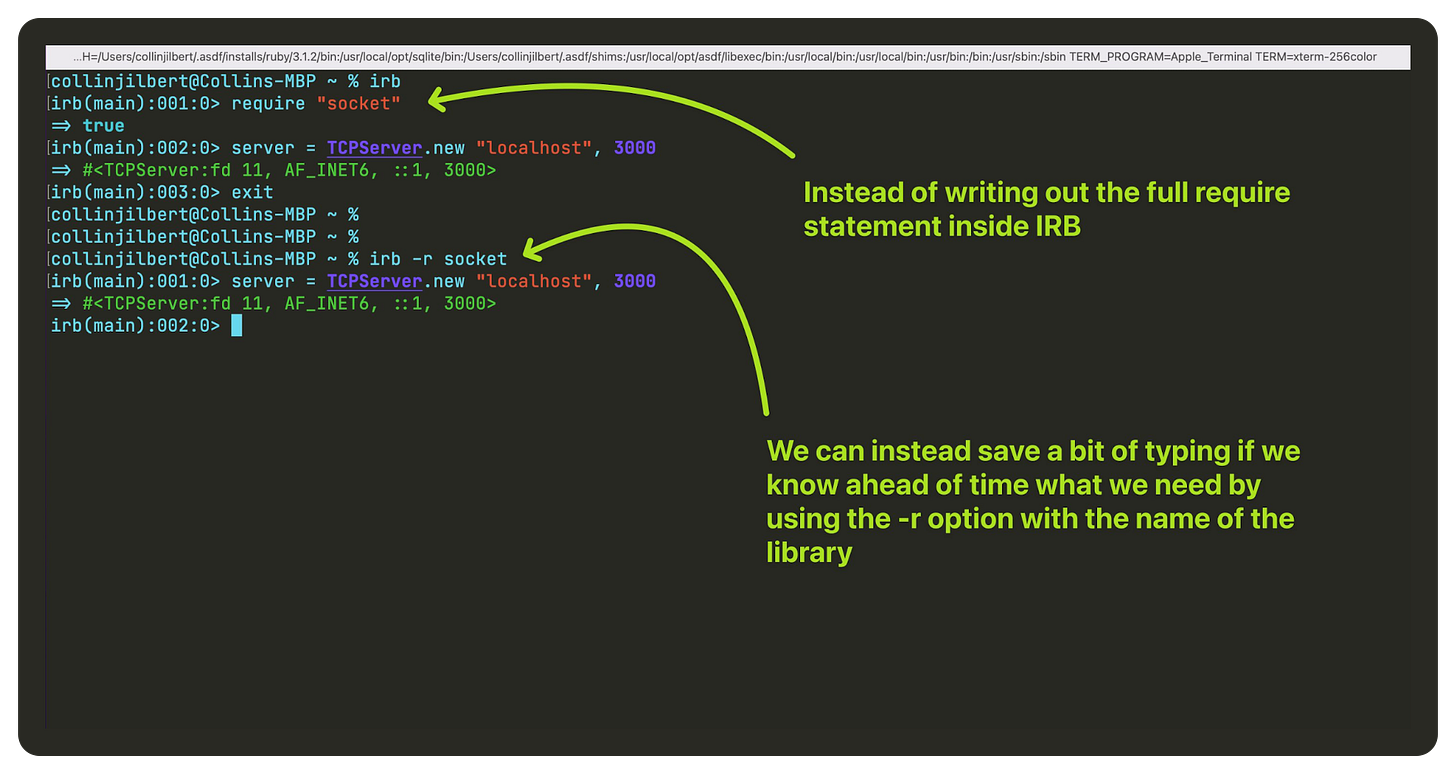
š Joe Masilotti shared a sample code showing how to add a hidden field to the button_to helper:
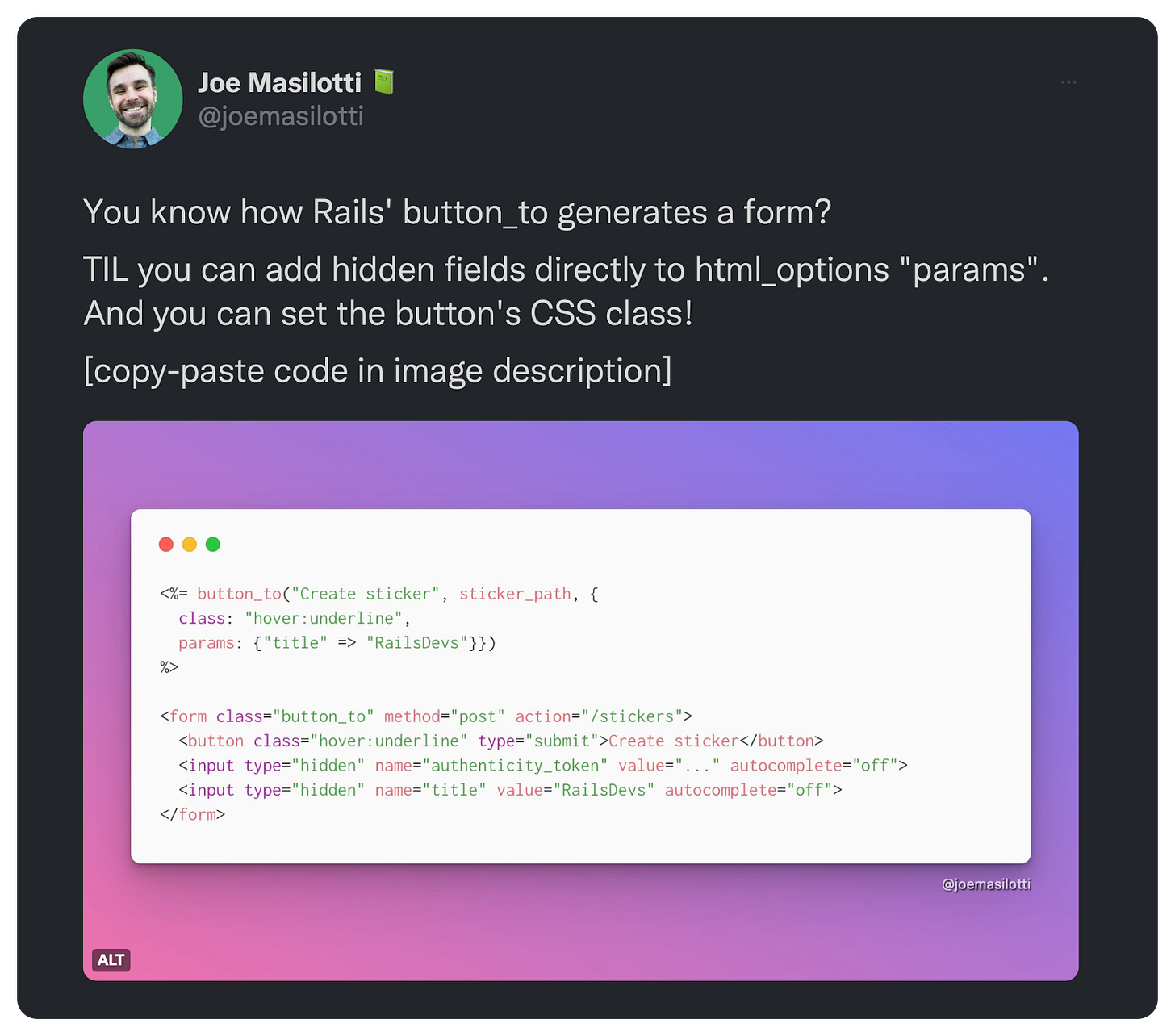
If you read so far and you like the content, maybe you take into consideration sharing this and subscribe:
Related (but not Ruby specific)
Vinicius Stock shared two VSCode tips for people working with Sorbet:
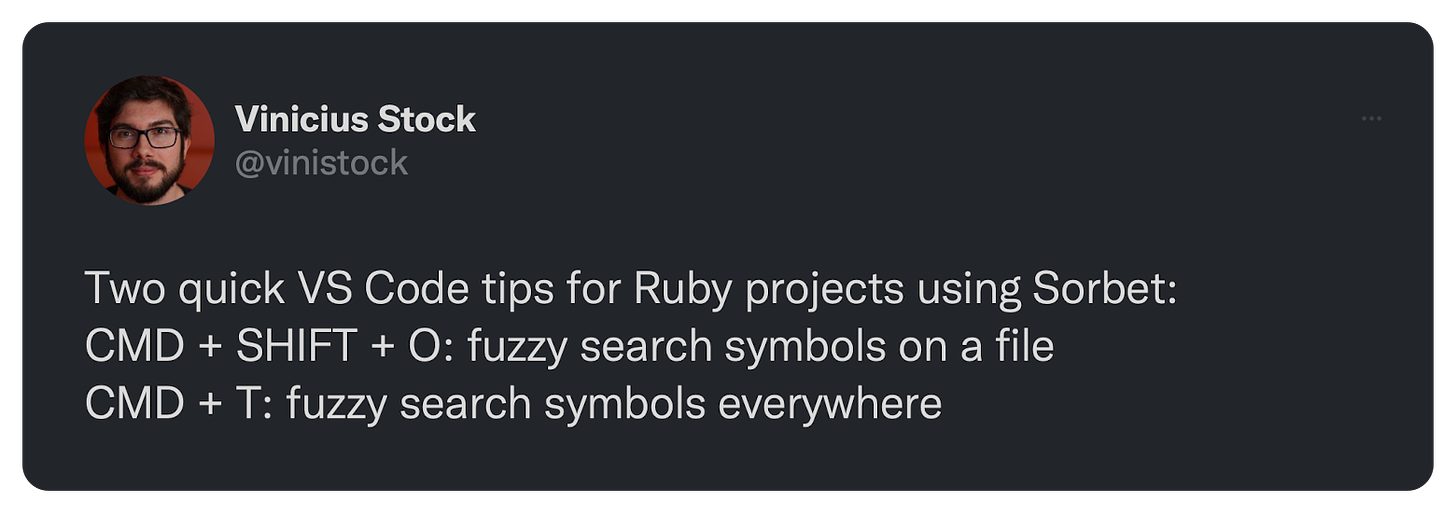
Joe Masilotti š shared asked about Ruby and Rails communities and he received a lot of replies:
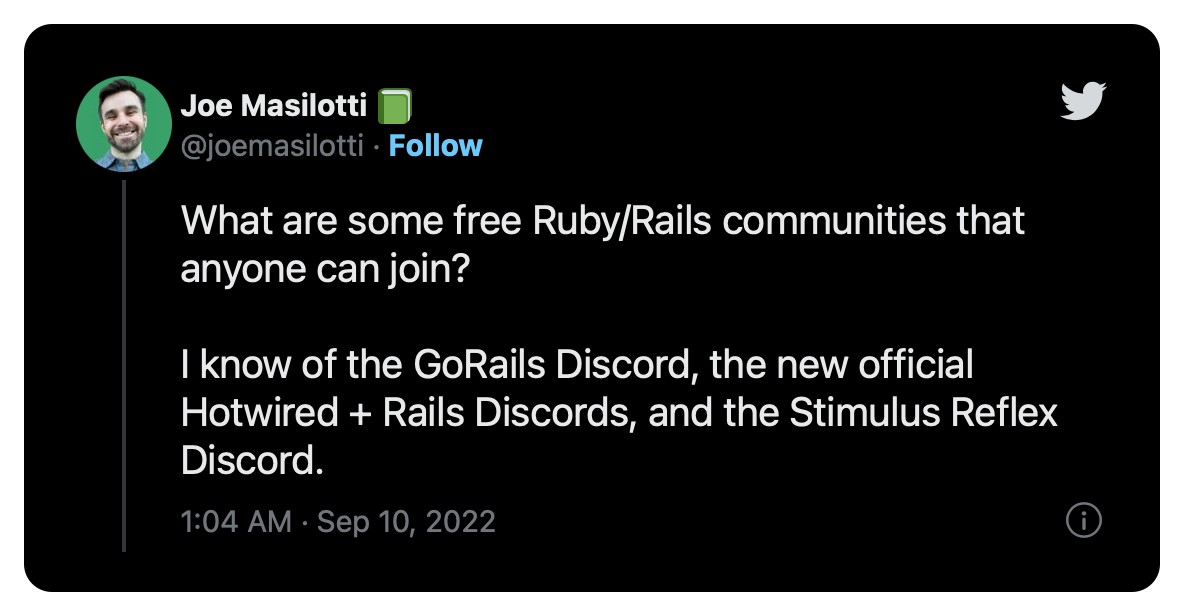
Here are some of the communities shared in the conversation:
Kasper helps your Ruby&Rails - on Discord
GoRails - on Discord
Hotwired - on Discord
Ruby on Rails - on Discord
Stimulus Reflex - on Discord
Avo - on Discord
Ruby on Rails - on Discourse
Ruby on Rails Link - on Slack
Ruby Lang - on Discord
Ruby on Rails - on Twitter Communities
Aprende RoR - on Discord
Official Ruby on Rails - on Discord
Ruby - on Discord
Bullet Train - on Discord
Bridgetown - on Discord
New projects built with Ruby
š¢ Julian Rubisch shared that they launched railsreviews.com - Reactive Rails Reviews.
š¢ Jeremy Smith shared launched railsinspire.com - A curated collection of code samples from Ruby on Rails projects.
Articles and Videos
RubyKaigi 2022 slides and more
Colby Swandale shared from his talk at RubyKaigi about Adding Type Signatures into Ruby Docs
Yusuke Endoh shared a Cookpad code puzzle that was created for RubyKaigi, but anyone can play it now: https://ruby-puzzles-2022.cookpad.tech
Jeremy Evans shared slides from RubyKaigi presentation: Fixing Assignment Evaluation Order
Koichi ITO shared their slides from RubyKaigi about Making Rubocop Super Fast
Takashi Kokubun shared their slides from RubyKaigi about Towards Ruby 4 JIT
Courses or Books
š Noel Rappin shared that the second version of his book Modern Front-end Developmen for Rails is now updated to work with Rails. Buy it here (PragmaticProgrammers shared on twitter also a discount code)
š David Colby shared that they made free their book Hotwired ATS, that is teaching how to build a complex Rails 7 application with Turbo, Stimulus, CableReady and Stimulus Reflex.
Something to read
Newsletters
Any Cable shared a new issue of monthly Any Cable updates: Any Cables Monthly #2 ā August, 2022
Greg Molnar shared a new edition of This Week in Rails created by Emmanuel Hayford.
Andrew Mason shared they released a new Ruby Radar issue #67 - New Rails Release!
Ruby Libhunt released a new version of their #329 Awesome Ruby newsletter.
Articles
Nate Berkopec shared an article written by Jemma Issroff about how to find faster in a sorted array using bsearch
Sam Ruby shared a good tutorial about how to use Turbo Stream and Stimulus with a Rails app on Fly
R7kamura shared about how to automate rubocop corrections on PRs.
The Ruby Dev shared installing Ruby on AWS EC2 with Cloud Formation
Something to watch š„ or listen š§
Videos
š„ Julian Rubisch shared a video showing how he is using CableReady::Updatable and Turbo forms to implement a previewable text area. See it ā Public Building RailsReviews - #1 Preview Component
š„ Vladimir Dementyev shared a new episode of AnyCasts where deploys AnyCable to Fly. See it ā Ep. 6: Learn to Fly.io with AnyCable
š„ Hexdevs shared a new episode of Open Source Thursdays about an introduction to how Faker works and how to contribute to it. See it ā Letās Faker Together: a Ruby library for generating fake data
š„ Drifting Ruby shared the video version of This Week in Rails. See eit ā This Week in Rails Sept 10th, 2022
Audio & Podcasts
š§ Drew Bragg shared a new episode about Coding Coders who Code with Ernesto. Listen ā Episode 10 - Ernesto Tagwerker
š§ Therubyonrailspodcast shared a new episode of The Ruby on Rails podcast about RubyConfMini: Listen ā Episode 434: All Things RubyConf Mini (Brittany + Jemma)
š§ Fullstack Ruby shared a new podcast about How to manage ruby apps dependencies. Listen ā Episode 6: How do you manage Ruby Application Dependencies
š§ Ruby For All shared a new episode about programming with ADHD. Listen ā Programming with ADHD
š§ JoĆ«l Quenneville shared a new episode of BikeShed with @EebsKobeissi. Listen ā 353: Mental Models
š§ Remote Ruby shared a new episode about the new Hatchbox.io V2. Listen ā Episode 196 ā The brand new Hatchbox.io V2
Gems, Libraries, and Updates
Aaron Patterson shared a release of Rack 3.0.0.rc1 and invited anyone to test it. Read the latest Changelog here.
Mike Dalessio shared the latest version of sqlite3 gem is out and it ships with precompiled sqlite. Read the Changelog here
Rob Sanheim shared a gem that helps running parallel_tests:
It will run parallel on multiple CPU cores. ParallelTests splits tests into even groups (by number of lines or runtime) and runs each group in a single process with its own database.
ęē¬ shared that Ruby 3.2 preview 2 is released. See the announcement here. Ruby 3.2 has WASI based WebAssembly support, Regexp timeout, YJIT arm64 support and many more things.
Hiroshi Shibata shared they released Ruby build with support doe Ruby 3.2.0 preview2. See the release
Koichi Ito shared rubocop performance 1.15. Check release notes
Konnor Rogers shared declarative shadow DOM components in Rails. See the code here.
Ruby Lib HuntĀ sharedĀ for creating chains, similar to chaining with then. The source code of this library is here. Here is a code sample:
Michael Nikitochkin shared a new version of the toxiproxy library that is a TCP proxy to simulate network and system conditions for chaos and resilience testing. See the project here and here is an example:
This was a long issue as there was a lot of great content that I discovered in Ruby community. I started to follow what is happening on Reddit communities so I will probably start bringing content from there.
Please consider sharing this on social media or with your colleagues: